AWT FlowLayout Class
Introduction
The class FlowLayout components in a left-to-right flow.Class declaration
Following is the declaration for java.awt.FlowLayout class:public class FlowLayout extends Object implements LayoutManager, Serializable
Field
Following are the fields for java.awt.BorderLayout class:- static int CENTER -- This value indicates that each row of components should be centered.
- static int LEADING -- This value indicates that each row of components should be justified to the leading edge of the container's orientation, for example, to the left in left-to-right orientations.
- static int LEFT -- This value indicates that each row of components should be left-justified.
- static int RIGHT -- This value indicates that each row of components should be right-justified.
- static int TRAILING -- This value indicates that each row of components should be justified to the trailing edge of the container's orientation, for example, to the right in left-to-right orientations.
Class constructors
S.N. | Constructor & Description |
---|---|
1 | FlowLayout() Constructs a new FlowLayout with a centered alignment and a default 5-unit horizontal and vertical gap. |
2 | FlowLayout(int align) Constructs a new FlowLayout with the specified alignment and a default 5-unit horizontal and vertical gap. |
3 | FlowLayout(int align, int hgap, int vgap) Creates a new flow layout manager with the indicated alignment and the indicated horizontal and vertical gaps. |
Class methods
S.N. | Method & Description |
---|---|
1 | void addLayoutComponent(String name, Component comp) Adds the specified component to the layout. |
2 | int getAlignment() Gets the alignment for this layout. |
3 | int getHgap() Gets the horizontal gap between components. |
4 | int getVgap() Gets the vertical gap between components. |
5 | void layoutContainer(Container target) Lays out the container. |
6 | Dimension minimumLayoutSize(Container target) Returns the minimum dimensions needed to layout the visible components contained in the specified target container. |
7 | Dimension preferredLayoutSize(Container target) Returns the preferred dimensions for this layout given the visible components in the specified target container. |
8 | void removeLayoutComponent(Component comp) Removes the specified component from the layout. |
9 | void setAlignment(int align) Sets the alignment for this layout. |
10 | void setHgap(int hgap) Sets the horizontal gap between components. |
11 | void setVgap(int vgap) Sets the vertical gap between components. |
12 | String toString() Returns a string representation of this FlowLayout object and its values. |
Methods inherited
This class inherits methods from the following classes:- java.lang.Object
FlowLayout Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtLayoutDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtLayoutDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; private Label msglabel; public AwtLayoutDemo(){ prepareGUI(); } public static void main(String[] args){ AwtLayoutDemo awtLayoutDemo = new AwtLayoutDemo(); awtLayoutDemo.showFlowLayoutDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); msglabel = new Label(); msglabel.setAlignment(Label.CENTER); msglabel.setText("Welcome to TutorialsPoint AWT Tutorial."); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showFlowLayoutDemo(){ headerLabel.setText("Layout in action: FlowLayout"); Panel panel = new Panel(); panel.setBackground(Color.darkGray); panel.setSize(200,200); FlowLayout layout = new FlowLayout(); layout.setHgap(10); layout.setVgap(10); panel.setLayout(layout); panel.add(new Button("OK")); panel.add(new Button("Cancel")); controlPanel.add(panel); mainFrame.setVisible(true); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtlayoutDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtlayoutDemoVerify the following output
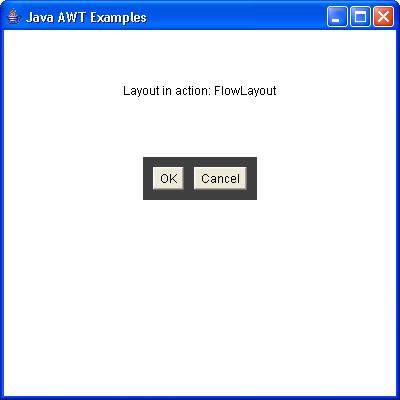
AWT GridLayout Class
Introduction
The class GridLayout arranges components in a rectangular grid.Class declaration
Following is the declaration for java.awt.GridLayout class:public class GridLayout extends Object implements LayoutManager, Serializable
Class constructors
S.N. | Constructor & Description |
---|---|
1 | GridLayout() Creates a grid layout with a default of one column per component, in a single row. |
2 | GridLayout(int rows, int cols) Creates a grid layout with the specified number of rows and columns. |
3 | GridLayout(int rows, int cols, int hgap, int vgap) Creates a grid layout with the specified number of rows and columns. |
Class methods
S.N. | Method & Description |
---|---|
1 | void addLayoutComponent(String name, Component comp) Adds the specified component with the specified name to the layout. |
2 | int getColumns() Gets the number of columns in this layout. |
3 | int getHgap() Gets the horizontal gap between components. |
4 | int getRows() Gets the number of rows in this layout. |
5 | int getVgap() Gets the vertical gap between components. |
6 | void layoutContainer(Container parent) Lays out the specified container using this layout. |
7 | Dimension minimumLayoutSize(Container parent) Determines the minimum size of the container argument using this grid layout. |
8 | Dimension preferredLayoutSize(Container parent) Determines the preferred size of the container argument using this grid layout. |
9 | void removeLayoutComponent(Component comp) Removes the specified component from the layout. |
10 | void setColumns(int cols) Sets the number of columns in this layout to the specified value. |
11 | void setHgap(int hgap) Sets the horizontal gap between components to the specified value. |
12 | void setRows(int rows) Sets the number of rows in this layout to the specified value. |
13 | void setVgap(int vgap) Sets the vertical gap between components to the specified value. |
14 | String toString() Returns the string representation of this grid layout's values. |
Methods inherited
This class inherits methods from the following classes:- java.lang.Object
GridLayout Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtLayoutDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtLayoutDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; private Label msglabel; public AwtLayoutDemo(){ prepareGUI(); } public static void main(String[] args){ AwtLayoutDemo awtLayoutDemo = new AwtLayoutDemo(); awtLayoutDemo.showGridLayoutDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); msglabel = new Label(); msglabel.setAlignment(Label.CENTER); msglabel.setText("Welcome to TutorialsPoint AWT Tutorial."); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showGridLayoutDemo(){ headerLabel.setText("Layout in action: GridLayout"); Panel panel = new Panel(); panel.setBackground(Color.darkGray); panel.setSize(300,300); GridLayout layout = new GridLayout(0,3); layout.setHgap(10); layout.setVgap(10); panel.setLayout(layout); panel.add(new Button("Button 1")); panel.add(new Button("Button 2")); panel.add(new Button("Button 3")); panel.add(new Button("Button 4")); panel.add(new Button("Button 5")); controlPanel.add(panel); mainFrame.setVisible(true); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtlayoutDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtlayoutDemoVerify the following output
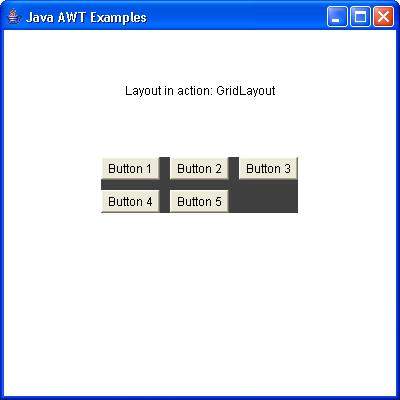
AWT GridBagLayout Class
Introduction
The class GridBagLayout arranges components in a horizontal and vertical manner.Class declaration
Following is the declaration for java.awt.GridBagLayout class:public class GridBagLayout extends Object implements LayoutManager2, Serializable
Field
Following are the fields for java.awt.BorderLayout class:- double[] columnWeights -- This field holds the overrides to the column weights.
- int[] columnWidths -- This field holds the overrides to the column minimum width.
- protected Hashtable comptable -- This hashtable maintains the association between a component and its gridbag constraints.
- protected GridBagConstraints defaultConstraints -- This field holds a gridbag constraints instance containing the default values, so if a component does not have gridbag constraints associated with it, then the component will be assigned a copy of the defaultConstraints.
- protected java.awt.GridBagLayoutInfo layoutInfo -- This field holds the layout information for the gridbag.
- protected static int MAXGRIDSIZE -- The maximum number of grid positions (both horizontally and vertically) that can be laid out by the grid bag layout.
- protected static int MINSIZE -- The smallest grid that can be laid out by the grid bag layout.
- protected static int PREFERREDSIZE -- The preferred grid size that can be laid out by the grid bag layout.
- int[] rowHeights -- This field holds the overrides to the row minimum heights.
- double[] rowWeights -- This field holds the overrides to the row weights.
Class constructors
S.N. | Constructor & Description |
---|---|
1 | GridBagLayout() Creates a grid bag layout manager. |
Class methods
S.N. | Method & Description |
---|---|
1 | void addLayoutComponent(Component comp, Object constraints) Adds the specified component to the layout, using the specified constraints object. |
2 | void addLayoutComponent(String name, Component comp) Adds the specified component with the specified name to the layout. |
3 | protected void adjustForGravity(GridBagConstraints constraints, Rectangle r) Adjusts the x, y, width, and height fields to the correct values depending on the constraint geometry and pads. |
4 | protected void AdjustForGravity(GridBagConstraints constraints, Rectangle r) This method is obsolete and supplied for backwards compatability only; new code should call adjustForGravity instead. |
5 | protected void arrangeGrid(Container parent) Lays out the grid. |
6 | protected void ArrangeGrid(Container parent) This method is obsolete and supplied for backwards compatability only; new code should call arrangeGrid instead. |
7 | GridBagConstraints getConstraints(Component comp) Gets the constraints for the specified component. |
8 | float getLayoutAlignmentX(Container parent) Returns the alignment along the x axis. |
9 | float getLayoutAlignmentY(Container parent) Returns the alignment along the y axis. |
10 | int[][] getLayoutDimensions() Determines column widths and row heights for the layout grid. |
11 | protected java.awt.GridBagLayoutInfo getLayoutInfo(Container parent, int sizeflag) Fills in an instance of GridBagLayoutInfo for the current set of managed children. |
12 | protected java.awt.GridBagLayoutInfo GetLayoutInfo(Container parent, int sizeflag) This method is obsolete and supplied for backwards compatability only; new code should call getLayoutInfo instead. |
13 | Point getLayoutOrigin() Determines the origin of the layout area, in the graphics coordinate space of the target container. |
14 | double[][] getLayoutWeights() Determines the weights of the layout grid's columns and rows. |
15 | protected Dimension getMinSize(Container parent, java.awt.GridBagLayoutInfo info) Figures out the minimum size of the master based on the information from getLayoutInfo(). |
16 | protected Dimension GetMinSize(Container parent, java.awt.GridBagLayoutInfo info) This method is obsolete and supplied for backwards compatability only; new code should call getMinSize instead. |
17 | void invalidateLayout(Container target) Invalidates the layout, indicating that if the layout manager has cached information it should be discarded. |
18 | void layoutContainer(Container parent) Lays out the specified container using this grid bag layout. |
19 | Point location(int x, int y) Determines which cell in the layout grid contains the point specified by (x, y). |
20 | protected GridBagConstraints lookupConstraints(Component comp) Retrieves the constraints for the specified component. |
21 | Dimension maximumLayoutSize(Container target) Returns the maximum dimensions for this layout given the components in the specified target container. |
22 | Dimension minimumLayoutSize(Container parent) Determines the minimum size of the parent container using this grid bag layout. |
23 | Dimension preferredLayoutSize(Container parent) Determines the preferred size of the parent container using this grid bag layout. |
24 | void removeLayoutComponent(Component comp) Removes the specified component from this layout. |
25 | void setConstraints(Component comp, GridBagConstraints constraints) Sets the constraints for the specified component in this layout. |
26 | String toString() Returns a string representation of this grid bag layout's values. |
Methods inherited
This class inherits methods from the following classes:- java.lang.Object
GridBagLayout Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtLayoutDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtLayoutDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; private Label msglabel; public AwtLayoutDemo(){ prepareGUI(); } public static void main(String[] args){ AwtLayoutDemo awtLayoutDemo = new AwtLayoutDemo(); awtLayoutDemo.showGridBagLayoutDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); msglabel = new Label(); msglabel.setAlignment(Label.CENTER); msglabel.setText("Welcome to TutorialsPoint AWT Tutorial."); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showGridBagLayoutDemo(){ headerLabel.setText("Layout in action: GridBagLayout"); Panel panel = new Panel(); panel.setBackground(Color.darkGray); panel.setSize(300,300); GridBagLayout layout = new GridBagLayout(); panel.setLayout(layout); GridBagConstraints gbc = new GridBagConstraints(); gbc.fill = GridBagConstraints.HORIZONTAL; gbc.gridx = 0; gbc.gridy = 0; panel.add(new Button("Button 1"),gbc); gbc.gridx = 1; gbc.gridy = 0; panel.add(new Button("Button 2"),gbc); gbc.fill = GridBagConstraints.HORIZONTAL; gbc.ipady = 20; gbc.gridx = 0; gbc.gridy = 1; panel.add(new Button("Button 3"),gbc); gbc.gridx = 1; gbc.gridy = 1; panel.add(new Button("Button 4"),gbc); gbc.gridx = 0; gbc.gridy = 2; gbc.fill = GridBagConstraints.HORIZONTAL; gbc.gridwidth = 2; panel.add(new Button("Button 5"),gbc); controlPanel.add(panel); mainFrame.setVisible(true); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtlayoutDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtlayoutDemoVerify the following output
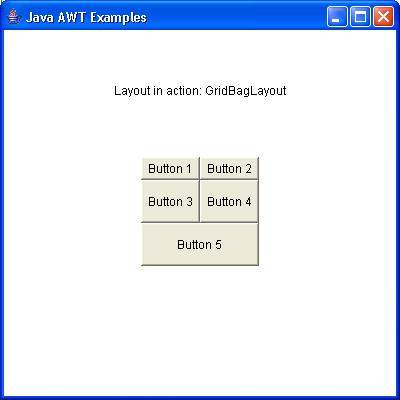
AWT Containers
Containers are integral part of AWT GUI components. A container provides a space where a component can be located. A Container in AWT is a component itself and it adds the capability to add component to itself. Following are noticable points to be considered.- Sub classes of Container are called as Containter. For example Panel, Frame and Window.
- Container can add only Component to itself.
- A default layout is present in each container which can be overridden using setLayout method.
AWT Container Class
Introduction
The class Container is the super class for the containers of AWT. Container object can contain other AWT components.Class declaration
Following is the declaration for java.awt.Container class:public class Container extends Component
Class constructors
S.N. | Constructor & Description |
---|---|
1 | Container() This creates a new Container. |
Class methods
S.N. | Method & Description |
---|---|
1 | Component add(Component comp) Appends the specified component to the end of this container. |
2 | Component add(Component comp, int index) Adds the specified component to this container at the given position. |
3 | void add(Component comp, Object constraints) Adds the specified component to the end of this container. |
4 | void add(Component comp, Object constraints, int index) Adds the specified component to this container with the specified constraints at the specified index. |
5 | Component add(String name, Component comp) Adds the specified component to this container. |
6 | void addContainerListener(ContainerListener l) Adds the specified container listener to receive container events from this container. |
7 | protected void addImpl(Component comp, Object constraints, int index) Adds the specified component to this container at the specified index. |
8 | void addNotify() Makes this Container displayable by connecting it to a native screen resource. |
9 | void addPropertyChangeListener(PropertyChangeListener listener) Adds a PropertyChangeListener to the listener list. |
10 | void addPropertyChangeListener(String propertyName, PropertyChangeListener listener) Adds a PropertyChangeListener to the listener list for a specific property. |
11 | void applyComponentOrientation(ComponentOrientation o) Sets the ComponentOrientation property of this container and all components contained within it. |
12 | boolean areFocusTraversalKeysSet(int id) Returns whether the Set of focus traversal keys for the given focus traversal operation has been explicitly defined for this Container. |
13 | int countComponents() Deprecated. As of JDK version 1.1, replaced by getComponentCount(). |
14 | void deliverEvent(Event e) Deprecated. As of JDK version 1.1, replaced by dispatchEvent(AWTEvent e) |
15 | void doLayout() Causes this container to lay out its components. |
16 | Component findComponentAt(int x, int y) Locates the visible child component that contains the specified position. |
17 | Component findComponentAt(Point p) Locates the visible child component that contains the specified point. |
18 | float getAlignmentX() Returns the alignment along the x axis. |
19 | float getAlignmentY() Returns the alignment along the y axis. |
20 | Component getComponent(int n) Gets the nth component in this container. |
21 | Component getComponentAt(int x, int y) Locates the component that contains the x,y position. |
22 | Component getComponentAt(Point p) Gets the component that contains the specified point. |
23 | int getComponentCount() Gets the number of components in this panel. |
24 | Component[] getComponents() Gets all the components in this container. |
25 | int getComponentZOrder(Component comp) Returns the z-order index of the component inside the container. |
26 | ContainerListener[] getContainerListeners() Returns an array of all the container listeners registered on this container. |
27 | Set<AWTKeyStroke> getFocusTraversalKeys(int id) Returns the Set of focus traversal keys for a given traversal operation for this Container. |
28 | FocusTraversalPolicy getFocusTraversalPolicy() Returns the focus traversal policy that will manage keyboard traversal of this Container's children, or null if this Container is not a focus cycle root. |
29 | Insets getInsets() Determines the insets of this container, which indicate the size of the container's border. |
30 | LayoutManager getLayout() Gets the layout manager for this container. |
31 | <T extends EventListener> T[] getListeners(Class<T> listenerType) Returns an array of all the objects currently registered as FooListeners upon this Container. |
32 | Dimension getMaximumSize() Returns the maximum size of this container. |
33 | Dimension getMinimumSize() Returns the minimum size of this container. |
34 | Point getMousePosition(boolean allowChildren) Returns the position of the mouse pointer in this Container's coordinate space if the Container is under the mouse pointer, otherwise returns null. |
35 | Dimension getPreferredSize() Returns the preferred size of this container. |
36 | Insets insets() Deprecated. As of JDK version 1.1, replaced by getInsets(). |
37 | void invalidate() Invalidates the container. |
38 | boolean isAncestorOf(Component c) Checks if the component is contained in the component hierarchy of this container. |
39 | boolean isFocusCycleRoot() Returns whether this Container is the root of a focus traversal cycle. |
40 | boolean isFocusCycleRoot(Container container) Returns whether the specified Container is the focus cycle root of this Container's focus traversal cycle. |
41 | boolean isFocusTraversalPolicyProvider() Returns whether this container provides focus traversal policy. |
42 | boolean isFocusTraversalPolicySet() Returns whether the focus traversal policy has been explicitly set for this Container. |
43 | void layout() Deprecated. As of JDK version 1.1, replaced by doLayout(). |
44 | void list(PrintStream out, int indent) Prints a listing of this container to the specified output stream. |
45 | void list(PrintWriter out, int indent) Prints out a list, starting at the specified indentation, to the specified print writer. |
46 | Component locate(int x, int y) Deprecated. As of JDK version 1.1, replaced by getComponentAt(int, int). |
47 | Dimension minimumSize() Deprecated. As of JDK version 1.1, replaced by getMinimumSize(). |
48 | void paint(Graphics g) Paints the container. |
49 | void paintComponents(Graphics g) Paints each of the components in this container. |
50 | protected String paramString() Returns a string representing the state of this Container. |
51 | Dimension preferredSize() Deprecated. As of JDK version 1.1, replaced by getPreferredSize(). |
52 | void print(Graphics g) Prints the container. |
53 | void printComponents(Graphics g) Prints each of the components in this container. |
54 | protected void processContainerEvent(ContainerEvent e) Processes container events occurring on this container by dispatching them to any registered ContainerListener objects. |
55 | protected void processEvent(AWTEvent e) Processes events on this container. |
56 | void remove(Component comp) Removes the specified component from this container. |
57 | void remove(int index) Removes the component, specified by index, from this container. |
58 | void removeAll() Removes all the components from this container. |
59 | void removeContainerListener(ContainerListener l) Removes the specified container listener so it no longer receives container events from this container. |
60 | void removeNotify() Makes this Container undisplayable by removing its connection to its native screen resource. |
61 | void setComponentZOrder(Component comp, int index) Moves the specified component to the specified z-order index in the container. |
62 | void setFocusCycleRoot(boolean focusCycleRoot) Sets whether this Container is the root of a focus traversal cycle. |
63 | void setFocusTraversalKeys(int id, Set<? extends AWTKeyStroke> keystrokes) Sets the focus traversal keys for a given traversal operation for this Container. |
64 | void setFocusTraversalPolicy(FocusTraversalPolicy policy) Sets the focus traversal policy that will manage keyboard traversal of this Container's children, if this Container is a focus cycle root. |
65 | void setFocusTraversalPolicyProvider(boolean provider) Sets whether this container will be used to provide focus traversal policy. |
66 | void setFont(Font f) Sets the font of this container. |
67 | void setLayout(LayoutManager mgr) Sets the layout manager for this container. |
68 | void transferFocusBackward() Transfers the focus to the previous component, as though this Component were the focus owner. |
69 | void transferFocusDownCycle() Transfers the focus down one focus traversal cycle. |
70 | void update(Graphics g) Updates the container. |
71 | void validate() Validates this container and all of its subcomponents. |
72 | protected void validateTree() Recursively descends the container tree and recomputes the layout for any subtrees marked as needing it (those marked as invalid). |
Methods inherited
This class inherits methods from the following classes:- java.awt.Component
- java.lang.Object
AWT Panel Class
Introduction
The class Panel is the simplest container class. It provides space in which an application can attach any other component, including other panels. It uses FlowLayout as default layout manager.Class declaration
Following is the declaration for java.awt.Panel class:public class Panel extends Container implements Accessible
Class constructors
S.N. | Constructor & Description |
---|---|
1 | Panel() Creates a new panel using the default layout manager. |
2 | Panel(LayoutManager layout) Creates a new panel with the specified layout manager. |
Class methods
S.N. | Method & Description |
---|---|
1 | void addNotify() Creates the Panel's peer. |
2 | AccessibleContext getAccessibleContext() Gets the AccessibleContext associated with this Panel. |
Methods inherited
This class inherits methods from the following classes:- java.awt.Container
- java.awt.Component
- java.lang.Object
Panel Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtContainerDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtContainerDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; private Label msglabel; public AwtContainerDemo(){ prepareGUI(); } public static void main(String[] args){ AwtContainerDemo awtContainerDemo = new AwtContainerDemo(); awtContainerDemo.showPanelDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); msglabel = new Label(); msglabel.setAlignment(Label.CENTER); msglabel.setText("Welcome to TutorialsPoint AWT Tutorial."); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showPanelDemo(){ headerLabel.setText("Container in action: Panel"); Panel panel = new Panel(); panel.setBackground(Color.magenta); panel.setLayout(new FlowLayout()); panel.add(msglabel); controlPanel.add(panel); mainFrame.setVisible(true); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtContainerDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtContainerDemoVerify the following output
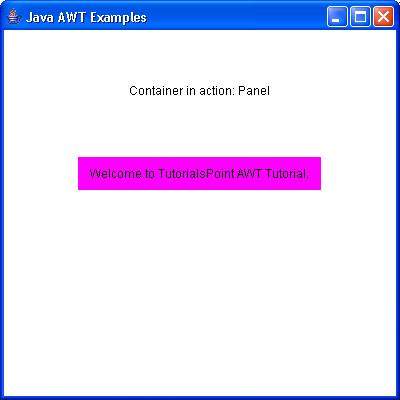
AWT Frame Class
Introduction
The class Frame is a top level window with border and title. It uses BorderLayout as default layout manager.Class declaration
Following is the declaration for java.awt.Frame class:public class Frame extends Window implements MenuContainer
Field
Following are the fields for java.awt.Frame class:- static float BOTTOM_ALIGNMENT -- Ease-of-use constant for getAlignmentY.
- static int CROSSHAIR_CURSOR -- Deprecated. replaced by Cursor.CROSSHAIR_CURSOR.
- static int DEFAULT_CURSOR -- Deprecated. replaced by Cursor.DEFAULT_CURSOR.
- static int E_RESIZE_CURSOR -- Deprecated. replaced by Cursor.E_RESIZE_CURSOR.
- static int HAND_CURSOR -- Deprecated. replaced by Cursor.HAND_CURSOR.
- static int ICONIFIED -- This state bit indicates that frame is iconified.
- static int MAXIMIZED_BOTH -- This state bit mask indicates that frame is fully maximized (that is both horizontally and vertically).
- static int MAXIMIZED_HORIZ -- This state bit indicates that frame is maximized in the horizontal direction.
- static int MAXIMIZED_VERT -- This state bit indicates that frame is maximized in the vertical direction.
- static int MOVE_CURSOR -- Deprecated. replaced by Cursor.MOVE_CURSOR.
- static int N_RESIZE_CURSOR -- Deprecated. replaced by Cursor.N_RESIZE_CURSOR.
- static int NE_RESIZE_CURSOR -- Deprecated. replaced by Cursor.NE_RESIZE_CURSOR.
- static int NORMAL -- Frame is in the "normal" state.
- static int NW_RESIZE_CURSOR -- Deprecated. replaced by Cursor.NW_RESIZE_CURSOR.
- static int S_RESIZE_CURSOR -- Deprecated. replaced by Cursor.S_RESIZE_CURSOR.
- static int SE_RESIZE_CURSOR -- Deprecated. replaced by Cursor.SE_RESIZE_CURSOR.
- static int SW_RESIZE_CURSOR -- Deprecated. replaced by Cursor.SW_RESIZE_CURSOR.
- static int TEXT_CURSOR -- Deprecated. replaced by Cursor.TEXT_CURSOR.
- static int W_RESIZE_CURSOR -- Deprecated. replaced by Cursor.W_RESIZE_CURSOR.
- static int WAIT_CURSOR -- Deprecated. replaced by Cursor.WAIT_CURSOR.
Class constructors
S.N. | Constructor & Description |
---|---|
1 | Frame() Constructs a new instance of Frame that is initially invisible. |
2 | Frame(GraphicsConfiguration gc) Constructs a new, initially invisible Frame with the specified GraphicsConfiguration. |
3 | Frame(String title) Constructs a new, initially invisible Frame object with the specified title. |
4 | Frame(String title, GraphicsConfiguration gc) Constructs a new, initially invisible Frame object with the specified title and a GraphicsConfiguration. |
Class methods
S.N. | Method & Description |
---|---|
1 | void addNotify() Makes this Frame displayable by connecting it to a native screen resource. |
2 | AccessibleContext getAccessibleContext() Gets the AccessibleContext associated with this Frame. |
3 | int getCursorType() Deprecated. As of JDK version 1.1, replaced by Component.getCursor(). |
4 | int getExtendedState() Gets the state of this frame. |
5 | static Frame[] getFrames() Returns an array of all Frames created by this application. |
6 | Image getIconImage() Returns the image to be displayed as the icon for this frame. |
7 | Rectangle getMaximizedBounds() Gets maximized bounds for this frame. |
8 | MenuBar getMenuBar() Gets the menu bar for this frame. |
9 | int getState() Gets the state of this frame (obsolete). |
10 | String getTitle() Gets the title of the frame. |
11 | boolean isResizable() Indicates whether this frame is resizable by the user. |
12 | boolean isUndecorated() Indicates whether this frame is undecorated. |
13 | protected String paramString() Returns a string representing the state of this Frame. |
14 | void remove(MenuComponent m) Removes the specified menu bar from this frame. |
15 | void removeNotify() Makes this Frame undisplayable by removing its connection to its native screen resource. |
16 | void setCursor(int cursorType) Deprecated. As of JDK version 1.1, replaced by Component.setCursor(Cursor). |
17 | void setExtendedState(int state) Sets the state of this frame. |
18 | void setIconImage(Image image) Sets the image to be displayed as the icon for this window. |
19 | void setMaximizedBounds(Rectangle bounds) Sets the maximized bounds for this frame. |
20 | void setMenuBar(MenuBar mb) Sets the menu bar for this frame to the specified menu bar. |
21 | void setResizable(boolean resizable) Sets whether this frame is resizable by the user. |
22 | void setState(int state) Sets the state of this frame (obsolete). |
23 | void setTitle(String title) Sets the title for this frame to the specified string. |
24 | void setUndecorated(boolean undecorated) Disables or enables decorations for this frame. |
Methods inherited
This class inherits methods from the following classes:- java.awt.Window
- java.awt.Container
- java.awt.Component
- java.lang.Object
Frame Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtContainerDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtContainerDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; private Label msglabel; public AwtContainerDemo(){ prepareGUI(); } public static void main(String[] args){ AwtContainerDemo awtContainerDemo = new AwtContainerDemo(); awtContainerDemo.showFrameDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); msglabel = new Label(); msglabel.setAlignment(Label.CENTER); msglabel.setText("Welcome to TutorialsPoint AWT Tutorial."); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showFrameDemo(){ headerLabel.setText("Container in action: Frame"); final Frame frame = new Frame(); frame.setSize(300, 300); frame.setLayout(new FlowLayout()); frame.add(msglabel); frame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ frame.dispose(); } }); Button okButton = new Button("Open a Frame"); okButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { statusLabel.setText("A Frame shown to the user."); frame.setVisible(true); } }); controlPanel.add(okButton); mainFrame.setVisible(true); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtContainerDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtContainerDemoVerify the following output
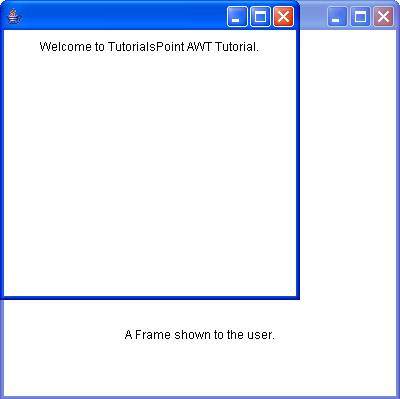
AWT Window Class
Introduction
The class Window is a top level window with no border and no menubar. It uses BorderLayout as default layout manager.Class declaration
Following is the declaration for java.awt.Window class:public class Window extends Container implements Accessible
Class constructors
S.N. | Constructor & Description |
---|---|
1 | Window(Frame owner) Constructs a new, initially invisible window with the specified Frame as its owner. |
2 | Window(Window owner) Constructs a new, initially invisible window with the specified Window as its owner. |
3 | Window(Window owner, GraphicsConfiguration gc) Constructs a new, initially invisible window with the specified owner Window and a GraphicsConfiguration of a screen device. |
Class methods
S.N. | Method & Description |
---|---|
1 | void addNotify() Makes this Window displayable by creating the connection to its native screen resource. |
2 | void addPropertyChangeListener(PropertyChangeListener listener) Adds a PropertyChangeListener to the listener list. |
3 | void addPropertyChangeListener(String propertyName, PropertyChangeListener listener) Adds a PropertyChangeListener to the listener list for a specific property. |
4 | void addWindowFocusListener(WindowFocusListener l) Adds the specified window focus listener to receive window events from this window. |
5 | void addWindowListener(WindowListener l) Adds the specified window listener to receive window events from this window. |
6 | void addWindowStateListener(WindowStateListener l) Adds the specified window state listener to receive window events from this window. |
7 | void applyResourceBundle(ResourceBundle rb) Deprecated. As of J2SE 1.4, replaced by Component.applyComponentOrientation. |
8 | void applyResourceBundle(String rbName) Deprecated. As of J2SE 1.4, replaced by Component.applyComponentOrientation. |
9 | void createBufferStrategy(int numBuffers) Creates a new strategy for multi-buffering on this component. |
10 | void createBufferStrategy(int numBuffers, BufferCapabilities caps) Creates a new strategy for multi-buffering on this component with the required buffer capabilities. |
11 | void dispose() Releases all of the native screen resources used by this Window, its subcomponents, and all of its owned children. |
12 | AccessibleContext getAccessibleContext() Gets the AccessibleContext associated with this Window. |
13 | BufferStrategy getBufferStrategy() Returns the BufferStrategy used by this component. |
14 | boolean getFocusableWindowState() Returns whether this Window can become the focused Window if it meets the other requirements outlined in isFocusableWindow. |
15 | Container getFocusCycleRootAncestor() Always returns null because Windows have no ancestors; they represent the top of the Component hierarchy. |
16 | Component getFocusOwner() Returns the child Component of this Window that has focus if this Window is focused; returns null otherwise. |
17 | Set<AWTKeyStroke> getFocusTraversalKeys(int id) Gets a focus traversal key for this Window. |
18 | GraphicsConfiguration getGraphicsConfiguration() This method returns the GraphicsConfiguration used by this Window. |
19 | List<Image> getIconImages() Returns the sequence of images to be displayed as the icon for this window. |
20 | InputContext getInputContext() Gets the input context for this window. |
21 | <T extends EventListener> T[] getListeners(Class<T> listenerType) Returns an array of all the objects currently registered as FooListeners upon this Window. |
22 | Locale getLocale() Gets the Locale object that is associated with this window, if the locale has been set. |
23 | Dialog.ModalExclusionType getModalExclusionType() Returns the modal exclusion type of this window. |
24 | Component getMostRecentFocusOwner() Returns the child Component of this Window that will receive the focus when this Window is focused. |
25 | Window[] getOwnedWindows() Return an array containing all the windows this window currently owns. |
26 | Window getOwner() Returns the owner of this window. |
27 | static Window[] getOwnerlessWindows() Returns an array of all Windows created by this application that have no owner. |
28 | Toolkit getToolkit() Returns the toolkit of this frame. |
29 | String getWarningString() Gets the warning string that is displayed with this window. |
30 | WindowFocusListener[] getWindowFocusListeners() Returns an array of all the window focus listeners registered on this window. |
31 | WindowListener[] getWindowListeners() Returns an array of all the window listeners registered on this window. |
32 | static Window[] getWindows() Returns an array of all Windows, both owned and ownerless, created by this application. |
33 | WindowStateListener[] getWindowStateListeners() Returns an array of all the window state listeners registered on this window. |
34 | void hide() Deprecated. As of JDK version 1.5, replaced by setVisible(boolean). |
35 | boolean isActive() Returns whether this Window is active. |
36 | boolean isAlwaysOnTop() Returns whether this window is an always-on-top window. |
37 | boolean isAlwaysOnTopSupported() Returns whether the always-on-top mode is supported for this window. |
38 | boolean isFocusableWindow() Returns whether this Window can become the focused Window, that is, whether this Window or any of its subcomponents can become the focus owner. |
39 | boolean isFocusCycleRoot() Always returns true because all Windows must be roots of a focus traversal cycle. |
40 | boolean isFocused() Returns whether this Window is focused. |
41 | boolean isLocationByPlatform() Returns true if this Window will appear at the default location for the native windowing system the next time this Window is made visible. |
42 | boolean isShowing() Checks if this Window is showing on screen. |
43 | void pack() Causes this Window to be sized to fit the preferred size and layouts of its subcomponents. |
44 | void paint(Graphics g) Paints the container. |
45 | boolean postEvent(Event e) Deprecated. As of JDK version 1.1 replaced by dispatchEvent(AWTEvent). |
46 | protected void processEvent(AWTEvent e) Processes events on this window. |
47 | protected void processWindowEvent(WindowEvent e) Processes window events occurring on this window by dispatching them to any registered WindowListener objects. |
48 | protected void processWindowFocusEvent(WindowEvent e) Processes window focus event occuring on this window by dispatching them to any registered WindowFocusListener objects. |
49 | protected void processWindowStateEvent(WindowEvent e) Processes window state event occuring on this window by dispatching them to any registered WindowStateListener objects. |
50 | void removeNotify() Makes this Container undisplayable by removing its connection to its native screen resource. |
51 | void removeWindowFocusListener(WindowFocusListener l) Removes the specified window focus listener so that it no longer receives window events from this window. |
52 | void removeWindowListener(WindowListener l) Removes the specified window listener so that it no longer receives window events from this window. |
53 | void removeWindowStateListener(WindowStateListener l) Removes the specified window state listener so that it no longer receives window events from this window. |
54 | void reshape(int x, int y, int width, int height) Deprecated. As of JDK version 1.1, replaced by setBounds(int, int, int, int). |
55 | void setAlwaysOnTop(boolean alwaysOnTop) Sets whether this window should always be above other windows. |
56 | void setBounds(int x, int y, int width, int height) Moves and resizes this component. |
57 | void setBounds(Rectangle r) Moves and resizes this component to conform to the new bounding rectangle r. |
58 | void setCursor(Cursor cursor) Set the cursor image to a specified cursor. |
59 | void setFocusableWindowState(boolean focusableWindowState) Sets whether this Window can become the focused Window if it meets the other requirements outlined in isFocusableWindow. |
60 | void setFocusCycleRoot(boolean focusCycleRoot) Does nothing because Windows must always be roots of a focus traversal cycle. |
61 | void setIconImage(Image image) Sets the image to be displayed as the icon for this window. |
62 | void setIconImages(List<? extends Image> icons) Sets the sequence of images to be displayed as the icon for this window. |
63 | void setLocationByPlatform(boolean locationByPlatform) Sets whether this Window should appear at the default location for the native windowing system or at the current location (returned by getLocation) the next time the Window is made visible. |
64 | void setLocationRelativeTo(Component c) Sets the location of the window relative to the specified component. |
65 | void setMinimumSize(Dimension minimumSize) Sets the minimum size of this window to a constant value. |
66 | void setModalExclusionType(Dialog.ModalExclusionType exclusionType) Specifies the modal exclusion type for this window. |
67 | void setSize(Dimension d) Resizes this component so that it has width d.width and height d.height. |
68 | void setSize(int width, int height) Resizes this component so that it has width width and height height. |
69 | void setVisible(boolean b) Shows or hides this Window depending on the value of parameter b. |
70 | void show() Deprecated. As of JDK version 1.5, replaced by setVisible(boolean). |
71 | void toBack() If this Window is visible, sends this Window to the back and may cause it to lose focus or activation if it is the focused or active Window. |
72 | void toFront() If this Window is visible, brings this Window to the front and may make it the focused Window. |
Methods inherited
This class inherits methods from the following classes:- java.awt.Window
- java.awt.Container
- java.awt.Component
- java.lang.Object
Window Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtContainerDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtContainerDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; private Label msglabel; public AwtContainerDemo(){ prepareGUI(); } public static void main(String[] args){ AwtContainerDemo awtContainerDemo = new AwtContainerDemo(); awtContainerDemo.showFrameDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); msglabel = new Label(); msglabel.setAlignment(Label.CENTER); msglabel.setText("Welcome to TutorialsPoint AWT Tutorial."); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showWindowDemo(){ headerLabel.setText("Container in action: Window"); final MessageWindow window = new MessageWindow(mainFrame, "Welcome to TutorialsPoint AWT Tutorial."); Button okButton = new Button("Open a Window"); okButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { window.setVisible(true); statusLabel.setText("A Window shown to the user."); } }); controlPanel.add(okButton); mainFrame.setVisible(true); } class MessageWindow extends Window{ private String message; public MessageWindow(Frame parent, String message) { super(parent); this.message = message; setSize(300, 300); setLocationRelativeTo(parent); setBackground(Color.gray); } public void paint(Graphics g) { super.paint(g); g.drawRect(0,0,getSize().width - 1,getSize().height - 1); g.drawString(message,50,150); } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtContainerDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtContainerDemoVerify the following output
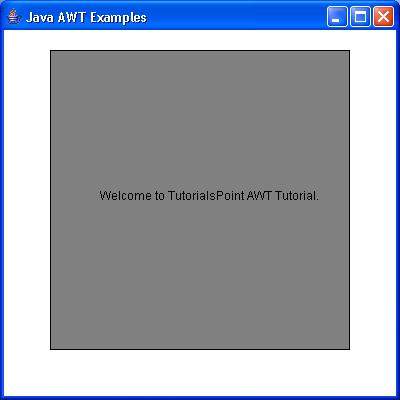
No comments:
Post a Comment