AWT MouseListener Interface
The class which processes the MouseEvent should implement this interface.The object of that class must be registered with a component. The object can be registered using the addMouseListener() method.Interface declaration
Following is the declaration for java.awt.event.MouseListener interface:public interface MouseListener extends EventListener
Interface methods
S.N. | Method & Description |
---|---|
1 | void mouseClicked(MouseEvent e) Invoked when the mouse button has been clicked (pressed and released) on a component. |
2 | void mouseEntered(MouseEvent e) Invoked when the mouse enters a component. |
3 | void mouseExited(MouseEvent e) Invoked when the mouse exits a component. |
4 | void mousePressed(MouseEvent e) Invoked when a mouse button has been pressed on a component. |
5 | void mouseReleased(MouseEvent e) Invoked when a mouse button has been released on a component. |
Methods inherited
This interface inherits methods from the following interfaces:- java.awt.EventListener
MouseListener Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtListenerDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtListenerDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtListenerDemo(){ prepareGUI(); } public static void main(String[] args){ AwtListenerDemo awtListenerDemo = new AwtListenerDemo(); awtListenerDemo.showMouseListenerDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showMouseListenerDemo(){ headerLabel.setText("Listener in action: MouseListener"); Panel panel = new Panel(); panel.setBackground(Color.magenta); panel.setLayout(new FlowLayout()); panel.addMouseListener(new CustomMouseListener()); Label msglabel = new Label(); msglabel.setAlignment(Label.CENTER); msglabel.setText("Welcome to TutorialsPoint AWT Tutorial."); msglabel.addMouseListener(new CustomMouseListener()); panel.add(msglabel); controlPanel.add(panel); mainFrame.setVisible(true); } class CustomMouseListener implements MouseListener{ public void mouseClicked(MouseEvent e) { statusLabel.setText("Mouse Clicked: (" +e.getX()+", "+e.getY() +")"); } public void mousePressed(MouseEvent e) { } public void mouseReleased(MouseEvent e) { } public void mouseEntered(MouseEvent e) { } public void mouseExited(MouseEvent e) { } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtListenerDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtListenerDemoVerify the following output
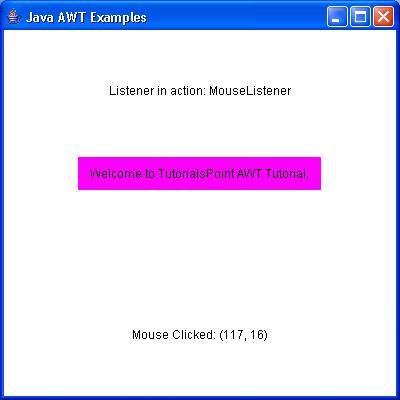
AWT TextListener Interface
The class which processes the TextEvent should implement this interface.The object of that class must be registered with a component. The object can be registered using the addTextListener() method.Interface declaration
Following is the declaration for java.awt.event.TextListener interface:public interface TextListener extends EventListener
Interface methods
S.N. | Method & Description |
---|---|
1 | void textValueChanged(TextEvent e) Invoked when the value of the text has changed. |
Methods inherited
This interface inherits methods from the following interfaces:- java.awt.EventListener
TextListener Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtListenerDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtListenerDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; private TextField textField; public AwtListenerDemo(){ prepareGUI(); } public static void main(String[] args){ AwtListenerDemo awtListenerDemo = new AwtListenerDemo(); awtListenerDemo.showTextListenerDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showTextListenerDemo(){ headerLabel.setText("Listener in action: TextListener"); textField = new TextField(10); textField.addTextListener(new CustomTextListener()); Button okButton = new Button("OK"); okButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { statusLabel.setText("Entered text: " + textField.getText()); } }); controlPanel.add(textField); controlPanel.add(okButton); mainFrame.setVisible(true); } class CustomTextListener implements TextListener { public void textValueChanged(TextEvent e) { statusLabel.setText("Entered text: " + textField.getText()); } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtListenerDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtListenerDemoVerify the following output
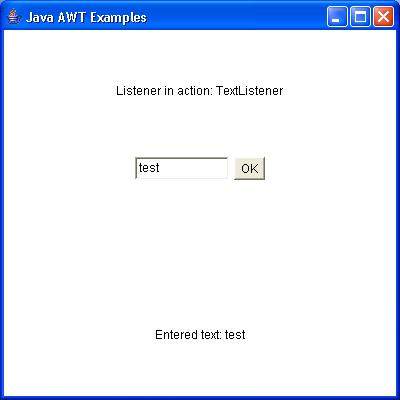
AWT WindowListener Interface
The class which processes the WindowEvent should implement this interface.The object of that class must be registered with a component. The object can be registered using the addWindowListener() method.Interface declaration
Following is the declaration for java.awt.event.WindowListener interface:public interface WindowListener extends EventListener
Interface methods
S.N. | Method & Description |
---|---|
1 | void windowActivated(WindowEvent e) Invoked when the Window is set to be the active Window. |
2 | void windowClosed(WindowEvent e) Invoked when a window has been closed as the result of calling dispose on the window. |
3 | void windowClosing(WindowEvent e) Invoked when the user attempts to close the window from the window's system menu. |
4 | void windowDeactivated(WindowEvent e) Invoked when a Window is no longer the active Window. |
5 | void windowDeiconified(WindowEvent e) Invoked when a window is changed from a minimized to a normal state. |
6 | void windowIconified(WindowEvent e) Invoked when a window is changed from a normal to a minimized state. |
7 | void windowOpened(WindowEvent e) Invoked the first time a window is made visible. |
Methods inherited
This interface inherits methods from the following interfaces:- java.awt.EventListener
WindowListener Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtListenerDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtListenerDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtListenerDemo(){ prepareGUI(); } public static void main(String[] args){ AwtListenerDemo awtListenerDemo = new AwtListenerDemo(); awtListenerDemo.showWindowListenerDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showWindowListenerDemo(){ headerLabel.setText("Listener in action: WindowListener"); Button okButton = new Button("OK"); aboutFrame = new Frame(); aboutFrame.setSize(300,200);; aboutFrame.setTitle("WindowListener Demo"); aboutFrame.addWindowListener(new CustomWindowListener()); Label msgLabel = new Label("Welcome to tutorialspoint."); msgLabel.setAlignment(Label.CENTER); msgLabel.setSize(100,100); aboutFrame.add(msgLabel); aboutFrame.setVisible(true); } class CustomWindowListener implements WindowListener { public void windowOpened(WindowEvent e) { } public void windowClosing(WindowEvent e) { aboutFrame.dispose(); } public void windowClosed(WindowEvent e) { } public void windowIconified(WindowEvent e) { } public void windowDeiconified(WindowEvent e) { } public void windowActivated(WindowEvent e) { } public void windowDeactivated(WindowEvent e) { } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtListenerDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtListenerDemoVerify the following output
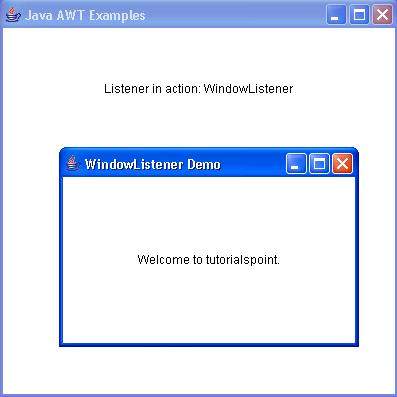
AWT AdjustmentListener Interface
Introduction
The interfaceAdjustmentListener is used for receiving adjustment events. The class that process adjustment events needs to implements this interface.Class declaration
Following is the declaration for java.awt.event.AdjustmentListener interface:public interface AdjustmentListener extends EventListener
Interface methods
S.N. | Method & Description |
---|---|
1 | void adjustmentValueChanged(AdjustmentEvent e) Invoked when the value of the adjustable has changed. |
Methods inherited
This class inherits methods from the following interfaces:- java.awt.event.EventListener
AdjustmentListener Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtListenerDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtListenerDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtListenerDemo(){ prepareGUI(); } public static void main(String[] args){ AwtListenerDemo awtListenerDemo = new AwtListenerDemo(); awtListenerDemo.showAdjustmentListenerDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showAdjustmentListenerDemo(){ headerLabel.setText("Listener in action: AdjustmentListener"); ScrollPane panel = new ScrollPane(); panel.setBackground(Color.magenta); panel.getHAdjustable().addAdjustmentListener(new CustomAdjustmentListener()); Label msglabel = new Label(); msglabel.setAlignment(Label.CENTER); msglabel.setText("Welcome to TutorialsPoint AWT Tutorial."); panel.add(msglabel); controlPanel.add(panel); mainFrame.setVisible(true); } class CustomAdjustmentListener implements AdjustmentListener { public void adjustmentValueChanged(AdjustmentEvent e) { statusLabel.setText("Adjustment value: "+Integer.toString(e.getValue())); } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtListenerDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtListenerDemoVerify the following output
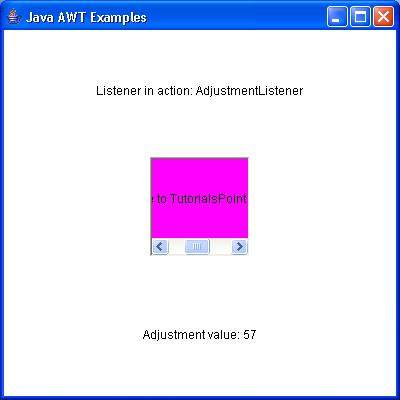
AWT ContainerListener Interface
Introduction
The interfaceContainerListener is used for receiving container events. The class that process container events needs to implements this interface.Class declaration
Following is the declaration for java.awt.event.ContainerListener interface:public interface ContainerListener extends EventListener
Interface methods
S.N. | Method & Description |
---|---|
1 | void componentAdded(ContainerEvent e) Invoked when a component has been added to the container. |
2 | void componentRemoved(ContainerEvent e) Invoked when a component has been removed from the container. |
Methods inherited
This class inherits methods from the following interfaces:- java.awt.event.EventListener
AdjustmentListener Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtListenerDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtListenerDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtListenerDemo(){ prepareGUI(); } public static void main(String[] args){ AwtListenerDemo awtListenerDemo = new AwtListenerDemo(); awtListenerDemo.showContainerListenerDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showContainerListenerDemo(){ headerLabel.setText("Listener in action: ContainerListener"); ScrollPane panel = new ScrollPane(); panel.setBackground(Color.magenta); panel.addContainerListener(new CustomContainerListener()); Label msglabel = new Label(); msglabel.setAlignment(Label.CENTER); msglabel.setText("Welcome to TutorialsPoint AWT Tutorial."); panel.add(msglabel); controlPanel.add(panel); mainFrame.setVisible(true); } class CustomContainerListener implements ContainerListener { public void componentAdded(ContainerEvent e) { statusLabel.setText(statusLabel.getText() + e.getComponent().getClass().getSimpleName() + " added. "); } public void componentRemoved(ContainerEvent e) { statusLabel.setText(statusLabel.getText() + e.getComponent().getClass().getSimpleName() + " removed. "); } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtListenerDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtListenerDemoVerify the following output
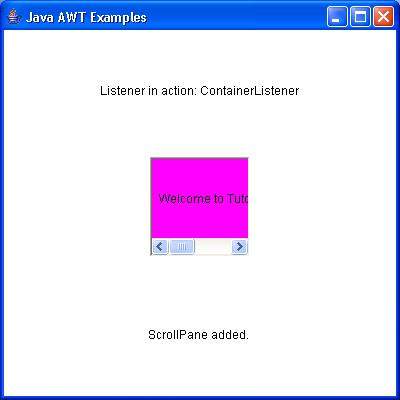
AWT MouseMotionListener Interface
Introduction
The interfaceMouseMotionListener is used for receiving mouse motion events on a component. The class that process mouse motion events needs to implements this interface.Class declaration
Following is the declaration for java.awt.event.MouseMotionListener interface:public interface MouseMotionListener extends EventListener
Interface methods
S.N. | Method & Description |
---|---|
1 | void mouseDragged(MouseEvent e) Invoked when a mouse button is pressed on a component and then dragged. |
2 | void mouseMoved(MouseEvent e) Invoked when the mouse cursor has been moved onto a component but no buttons have been pushed. |
Methods inherited
This class inherits methods from the following interfaces:- java.awt.event.EventListener
MouseMotionListener Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtListenerDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtListenerDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtListenerDemo(){ prepareGUI(); } public static void main(String[] args){ AwtListenerDemo awtListenerDemo = new AwtListenerDemo(); awtListenerDemo.showMouseMotionListenerDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showMouseMotionListenerDemo(){ headerLabel.setText("Listener in action: MouseMotionListener"); Panel panel = new Panel(); panel.setBackground(Color.magenta); panel.setLayout(new FlowLayout()); panel.addMouseMotionListener(new CustomMouseMotionListener()); Label msglabel = new Label(); msglabel.setAlignment(Label.CENTER); msglabel.setText("Welcome to TutorialsPoint AWT Tutorial."); panel.add(msglabel); controlPanel.add(panel); mainFrame.setVisible(true); } class CustomMouseMotionListener implements MouseMotionListener { public void mouseDragged(MouseEvent e) { statusLabel.setText("Mouse Dragged: ("+e.getX()+", "+e.getY() +")"); } public void mouseMoved(MouseEvent e) { statusLabel.setText("Mouse Moved: ("+e.getX()+", "+e.getY() +")"); } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtListenerDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtListenerDemoVerify the following output
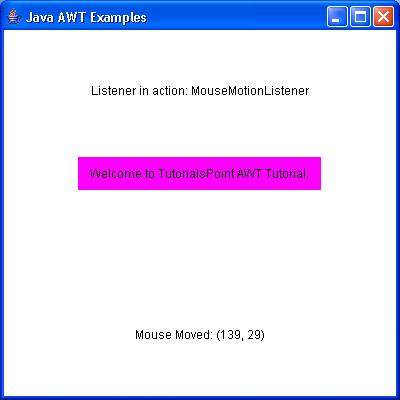
AWT FocusListener Interface
Introduction
The interfaceFocusListener is used for receiving keyboard focus events. The class that process focus events needs to implements this interface.Class declaration
Following is the declaration for java.awt.event.FocusListener interface:public interface FocusListener extends EventListener
Interface methods
S.N. | Method & Description |
---|---|
1 | void focusGained(FocusEvent e) Invoked when a component gains the keyboard focus. |
2 | void focusLost(FocusEvent e) Invoked when a component loses the keyboard focus. |
Methods inherited
This class inherits methods from the following interfaces:- java.awt.event.EventListener
FocusListener Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtListenerDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtListenerDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtListenerDemo(){ prepareGUI(); } public static void main(String[] args){ AwtListenerDemo awtListenerDemo = new AwtListenerDemo(); awtListenerDemo.showFocusListenerDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showFocusListenerDemo(){ headerLabel.setText("Listener in action: FocusListener"); Button okButton = new Button("OK"); Button cancelButton = new Button("Cancel"); okButton.addFocusListener(new CustomFocusListener()); cancelButton.addFocusListener(new CustomFocusListener()); controlPanel.add(okButton); controlPanel.add(cancelButton); mainFrame.setVisible(true); } class CustomFocusListener implements FocusListener{ public void focusGained(FocusEvent e) { statusLabel.setText(statusLabel.getText() + e.getComponent().getClass().getSimpleName() + " gained focus. "); } public void focusLost(FocusEvent e) { statusLabel.setText(statusLabel.getText() + e.getComponent().getClass().getSimpleName() + " lost focus. "); } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtListenerDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtListenerDemoVerify the following output
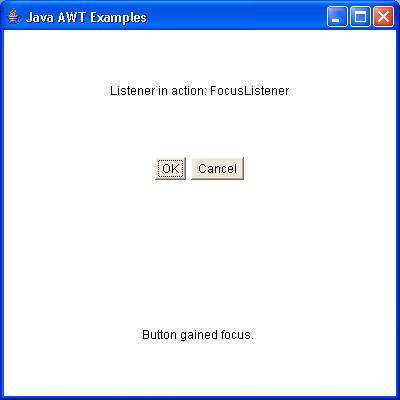
AWT Event Adapters
Adapters are abstract classes for receiving various events. The methods in these classes are empty. These classes exists as convenience for creating listener objects.AWT Adapters:
Following is the list of commonly used adapters while listening GUI events in AWT.AWT FocusAdapter Class
Introduction
The class FocusAdapter is an abstract (adapter) class for receiving keyboard focus events. All methods of this class are empty. This class is convenience class for creating listener objects.Class declaration
Following is the declaration for java.awt.event.FocusAdapter class:public abstract class FocusAdapter extends Object implements FocusListener
Class constructors
S.N. | Constructor & Description |
---|---|
1 | FocusAdapter() |
Class methods
S.N. | Method & Description |
---|---|
1 | void focusGained(FocusEvent e) Invoked when a component gains the keyboard focus. |
2 | focusLost(FocusEvent e) Invoked when a component loses the keyboard focus. |
Methods inherited
This class inherits methods from the following classes:- java.lang.Object
FocusAdapter Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtAdapterDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtAdapterDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtAdapterDemo(){ prepareGUI(); } public static void main(String[] args){ AwtAdapterDemo awtAdapterDemo = new AwtAdapterDemo(); awtAdapterDemo.showFocusAdapterDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showFocusAdapterDemo(){ headerLabel.setText("Listener in action: FocusAdapter"); Button okButton = new Button("OK"); Button cancelButton = new Button("Cancel"); okButton.addFocusListener(new FocusAdapter(){ public void focusGained(FocusEvent e) { statusLabel.setText(statusLabel.getText() + e.getComponent().getClass().getSimpleName() + " gained focus. "); } }); cancelButton.addFocusListener(new FocusAdapter(){ public void focusLost(FocusEvent e) { statusLabel.setText(statusLabel.getText() + e.getComponent().getClass().getSimpleName() + " lost focus. "); } }); controlPanel.add(okButton); controlPanel.add(cancelButton); mainFrame.setVisible(true); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtAdapterDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtAdapterDemoVerify the following output
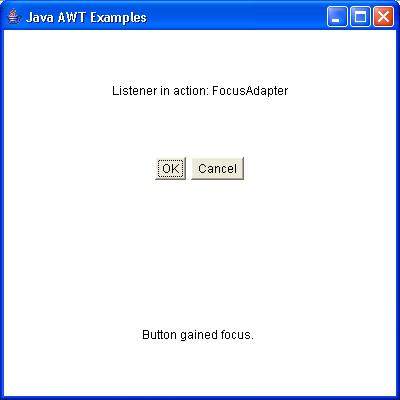
AWT KeyAdapter Class
Introduction
The class KeyAdapter is an abstract (adapter) class for receiving keyboard events. All methods of this class are empty. This class is convenience class for creating listener objects.Class declaration
Following is the declaration for java.awt.event.KeyAdapter class:public abstract class KeyAdapter extends Object implements KeyListener
Class constructors
S.N. | Constructor & Description |
---|---|
1 | KeyAdapter() |
Class methods
S.N. | Method & Description |
---|---|
1 | void keyPressed(KeyEvent e) Invoked when a key has been pressed. |
2 | void keyReleased(KeyEvent e) Invoked when a key has been released. |
3 | void keyTyped(KeyEvent e) Invoked when a key has been typed. |
Methods inherited
This class inherits methods from the following classes:- java.lang.Object
KeyAdapter Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtAdapterDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtAdapterDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtAdapterDemo(){ prepareGUI(); } public static void main(String[] args){ AwtAdapterDemo awtAdapterDemo = new AwtAdapterDemo(); awtAdapterDemo.showKeyAdapterDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showKeyAdapterDemo(){ headerLabel.setText("Listener in action: KeyAdapter"); final TextField textField = new TextField(10); textField.addKeyListener(new KeyAdapter() { public void keyPressed(KeyEvent e) { if(e.getKeyCode() == KeyEvent.VK_ENTER){ statusLabel.setText("Entered text: " + textField.getText()); } } }); Button okButton = new Button("OK"); okButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { statusLabel.setText("Entered text: " + textField.getText()); } }); controlPanel.add(textField); controlPanel.add(okButton); mainFrame.setVisible(true); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtAdapterDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtAdapterDemoVerify the following output
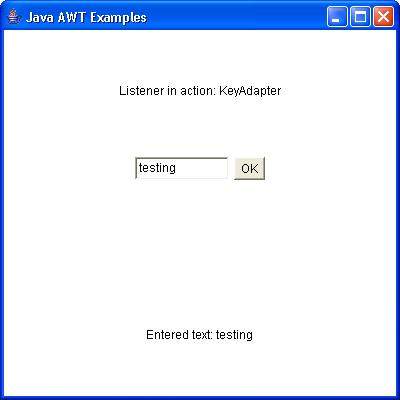
No comments:
Post a Comment