AWT Menu Classes
As we know that every top-level window has a menu bar associated with it. This menu bar consist of various menu choices available to the end user. Further each choice contains list of options which is called drop down menus. Menu and MenuItem controls are subclass of MenuComponent class.Menu Hiearchy
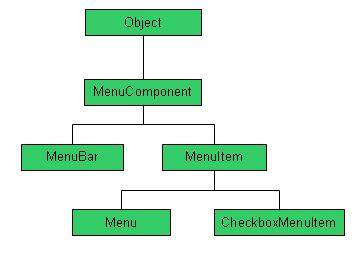
AWT MenuComponent Class
Introduction
MenuComponent is an abstract class and is the superclass for all menu-related components.Class declaration
Following is the declaration for java.awt.MenuComponent class:public abstract class MenuComponent extends Object implements Serializable
Class constructors
S.N. | Constructor & Description |
---|---|
1 | MenuComponent() Creates a MenuComponent. |
Class methods
void dispatchEvent(AWTEvent e)S.N. | Method & Description |
---|---|
1 | AccessibleContext getAccessibleContext() Gets the AccessibleContext associated with this MenuComponent. |
2 | Font getFont() Gets the font used for this menu component. |
3 | String getName() Gets the name of the menu component. |
4 | MenuContainer getParent() Returns the parent container for this menu component. |
5 | java.awt.peer.MenuComponentPeer getPeer() Deprecated. As of JDK version 1.1, programs should not directly manipulate peers. |
6 | protected Object getTreeLock() Gets this component's locking object (the object that owns the thread sychronization monitor) for AWT component-tree and layout operations. |
7 | protected String paramString() Returns a string representing the state of this MenuComponent. |
8 | boolean postEvent(Event evt) Deprecated. As of JDK version 1.1, replaced by dispatchEvent. |
9 | protected void processEvent(AWTEvent e) Processes events occurring on this menu component. |
10 | void removeNotify() Removes the menu component's peer. |
11 | void setFont(Font f) Sets the font to be used for this menu component to the specified font. |
12 | void setName(String name) Sets the name of the component to the specified string. |
13 | String toString() Returns a representation of this menu component as a string. |
Methods inherited
This class inherits methods from the following classes:- java.lang.Object
AWT MenuBar Class
Introduction
The MenuBar class provides menu bar bound to a frame and is platform specific.Class declaration
Following is the declaration for java.awt.MenuBar class:public class MenuBar extends MenuComponent implements MenuContainer, Accessible
Class constructors
S.N. | Constructor & Description |
---|---|
1 | MenuBar() Creates a new menu bar. |
Class methods
S.N. | Method & Description |
---|---|
1 | void dispatchEvent(AWTEvent e) |
2 | Menu add(Menu m) Adds the specified menu to the menu bar. |
3 | void addNotify() Creates the menu bar's peer. |
4 | int countMenus() Deprecated. As of JDK version 1.1, replaced by getMenuCount(). |
5 | void deleteShortcut(MenuShortcut s) Deletes the specified menu shortcut. |
6 | AccessibleContext getAccessibleContext() Gets the AccessibleContext associated with this MenuBar. |
7 | Menu getHelpMenu() Gets the help menu on the menu bar. |
8 | Menu getMenu(int i) Gets the specified menu. |
9 | int getMenuCount() Gets the number of menus on the menu bar. |
10 | MenuItem getShortcutMenuItem(MenuShortcut s) Gets the instance of MenuItem associated with the specified MenuShortcut object, or null if none of the menu items being managed by this menu bar is associated with the specified menu shortcut. |
11 | void remove(int index) Removes the menu located at the specified index from this menu bar. |
12 | void remove(MenuComponent m) Removes the specified menu component from this menu bar. |
13 | void removeNotify() Removes the menu bar's peer. |
14 | void setHelpMenu(Menu m) Sets the specified menu to be this menu bar's help menu. |
15 | Enumeration shortcuts() Gets an enumeration of all menu shortcuts this menu bar is managing. |
Methods inherited
This class inherits methods from the following classes:- java.awt.MenuComponent
- java.lang.Object
MenuBar Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AWTMenuDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AWTMenuDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AWTMenuDemo(){ prepareGUI(); } public static void main(String[] args){ AWTMenuDemo awtMenuDemo = new AWTMenuDemo(); awtMenuDemo.showMenuDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showMenuDemo(){ //create a menu bar final MenuBar menuBar = new MenuBar(); //create menus Menu fileMenu = new Menu("File"); Menu editMenu = new Menu("Edit"); final Menu aboutMenu = new Menu("About"); //create menu items MenuItem newMenuItem = new MenuItem("New",new MenuShortcut(KeyEvent.VK_N)); newMenuItem.setActionCommand("New"); MenuItem openMenuItem = new MenuItem("Open"); openMenuItem.setActionCommand("Open"); MenuItem saveMenuItem = new MenuItem("Save"); saveMenuItem.setActionCommand("Save"); MenuItem exitMenuItem = new MenuItem("Exit"); exitMenuItem.setActionCommand("Exit"); MenuItem cutMenuItem = new MenuItem("Cut"); cutMenuItem.setActionCommand("Cut"); MenuItem copyMenuItem = new MenuItem("Copy"); copyMenuItem.setActionCommand("Copy"); MenuItem pasteMenuItem = new MenuItem("Paste"); pasteMenuItem.setActionCommand("Paste"); MenuItemListener menuItemListener = new MenuItemListener(); newMenuItem.addActionListener(menuItemListener); openMenuItem.addActionListener(menuItemListener); saveMenuItem.addActionListener(menuItemListener); exitMenuItem.addActionListener(menuItemListener); cutMenuItem.addActionListener(menuItemListener); copyMenuItem.addActionListener(menuItemListener); pasteMenuItem.addActionListener(menuItemListener); final CheckboxMenuItem showWindowMenu = new CheckboxMenuItem("Show About", true); showWindowMenu.addItemListener(new ItemListener() { public void itemStateChanged(ItemEvent e) { if(showWindowMenu.getState()){ menuBar.add(aboutMenu); }else{ menuBar.remove(aboutMenu); } } }); //add menu items to menus fileMenu.add(newMenuItem); fileMenu.add(openMenuItem); fileMenu.add(saveMenuItem); fileMenu.addSeparator(); fileMenu.add(showWindowMenu); fileMenu.addSeparator(); fileMenu.add(exitMenuItem); editMenu.add(cutMenuItem); editMenu.add(copyMenuItem); editMenu.add(pasteMenuItem); //add menu to menubar menuBar.add(fileMenu); menuBar.add(editMenu); menuBar.add(aboutMenu); //add menubar to the frame mainFrame.setMenuBar(menuBar); mainFrame.setVisible(true); } class MenuItemListener implements ActionListener { public void actionPerformed(ActionEvent e) { statusLabel.setText(e.getActionCommand() + " MenuItem clicked."); } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AWTMenuDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AWTMenuDemoVerify the following output
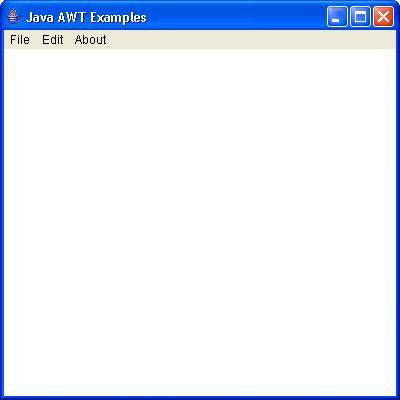
AWT MenuItem Class
Introduction
The MenuBar class represents the actual item in a menu. All items in a menu should derive from class MenuItem, or one of its subclasses. By default, it embodies a simple labeled menu item.Class declaration
Following is the declaration for java.awt.MenuItem class:public class MenuItem extends MenuComponent implements Accessible
Class constructors
S.N. | Constructor & Description |
---|---|
1 | MenuItem() Constructs a new MenuItem with an empty label and no keyboard shortcut. |
2 | MenuItem(String label) Constructs a new MenuItem with the specified label and no keyboard shortcut. |
3 | MenuItem(String label, MenuShortcut s) Create a menu item with an associated keyboard shortcut. |
Class methods
S.N. | Method & Description |
---|---|
1 | void addActionListener(ActionListener l) Adds the specified action listener to receive action events from this menu item. |
2 | void addNotify() Creates the menu item's peer. |
3 | void deleteShortcut() Delete any MenuShortcut object associated with this menu item. |
4 | void disable() Deprecated. As of JDK version 1.1, replaced by setEnabled(boolean). |
5 | protected void disableEvents(long eventsToDisable) Disables event delivery to this menu item for events defined by the specified event mask parameter. |
6 | void enable() Deprecated. As of JDK version 1.1, replaced by setEnabled(boolean). |
7 | void enable(boolean b) Deprecated. As of JDK version 1.1, replaced by setEnabled(boolean). |
8 | protected void enableEvents(long eventsToEnable) Enables event delivery to this menu item for events to be defined by the specified event mask parameter. |
9 | AccessibleContext getAccessibleContext() Gets the AccessibleContext associated with this MenuItem. |
10 | String getActionCommand() Gets the command name of the action event that is fired by this menu item. |
11 | ActionListener[] getActionListeners() Returns an array of all the action listeners registered on this menu item. |
12 | String getLabel() Gets the label for this menu item. |
13 | EventListener[] getListeners(Class listenerType) Returns an array of all the objects currently registered as FooListeners upon this MenuItem. |
14 | MenuShortcut getShortcut() Get the MenuShortcut object associated with this menu item. |
15 | boolean isEnabled() Checks whether this menu item is enabled. |
16 | String paramString() Returns a string representing the state of this MenuItem. |
17 | protected void processActionEvent(ActionEvent e) Processes action events occurring on this menu item, by dispatching them to any registered ActionListener objects. |
18 | protected void processEvent(AWTEvent e) Processes events on this menu item. |
19 | void removeActionListener(ActionListener l) Removes the specified action listener so it no longer receives action events from this menu item. |
20 | void setActionCommand(String command) Sets the command name of the action event that is fired by this menu item. |
21 | void setEnabled(boolean b) Sets whether or not this menu item can be chosen. |
22 | void setLabel(String label) Sets the label for this menu item to the specified label. |
23 | void setShortcut(MenuShortcut s) Set the MenuShortcut object associated with this menu item. |
Methods inherited
This class inherits methods from the following classes:- java.awt.MenuComponent
- java.lang.Object
MenuItem Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AWTMenuDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AWTMenuDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AWTMenuDemo(){ prepareGUI(); } public static void main(String[] args){ AWTMenuDemo awtMenuDemo = new AWTMenuDemo(); awtMenuDemo.showMenuDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showMenuDemo(){ //create a menu bar final MenuBar menuBar = new MenuBar(); //create menus Menu fileMenu = new Menu("File"); Menu editMenu = new Menu("Edit"); final Menu aboutMenu = new Menu("About"); //create menu items MenuItem newMenuItem = new MenuItem("New",new MenuShortcut(KeyEvent.VK_N)); newMenuItem.setActionCommand("New"); MenuItem openMenuItem = new MenuItem("Open"); openMenuItem.setActionCommand("Open"); MenuItem saveMenuItem = new MenuItem("Save"); saveMenuItem.setActionCommand("Save"); MenuItem exitMenuItem = new MenuItem("Exit"); exitMenuItem.setActionCommand("Exit"); MenuItem cutMenuItem = new MenuItem("Cut"); cutMenuItem.setActionCommand("Cut"); MenuItem copyMenuItem = new MenuItem("Copy"); copyMenuItem.setActionCommand("Copy"); MenuItem pasteMenuItem = new MenuItem("Paste"); pasteMenuItem.setActionCommand("Paste"); MenuItemListener menuItemListener = new MenuItemListener(); newMenuItem.addActionListener(menuItemListener); openMenuItem.addActionListener(menuItemListener); saveMenuItem.addActionListener(menuItemListener); exitMenuItem.addActionListener(menuItemListener); cutMenuItem.addActionListener(menuItemListener); copyMenuItem.addActionListener(menuItemListener); pasteMenuItem.addActionListener(menuItemListener); final CheckboxMenuItem showWindowMenu = new CheckboxMenuItem("Show About", true); showWindowMenu.addItemListener(new ItemListener() { public void itemStateChanged(ItemEvent e) { if(showWindowMenu.getState()){ menuBar.add(aboutMenu); }else{ menuBar.remove(aboutMenu); } } }); //add menu items to menus fileMenu.add(newMenuItem); fileMenu.add(openMenuItem); fileMenu.add(saveMenuItem); fileMenu.addSeparator(); fileMenu.add(showWindowMenu); fileMenu.addSeparator(); fileMenu.add(exitMenuItem); editMenu.add(cutMenuItem); editMenu.add(copyMenuItem); editMenu.add(pasteMenuItem); //add menu to menubar menuBar.add(fileMenu); menuBar.add(editMenu); menuBar.add(aboutMenu); //add menubar to the frame mainFrame.setMenuBar(menuBar); mainFrame.setVisible(true); } class MenuItemListener implements ActionListener { public void actionPerformed(ActionEvent e) { statusLabel.setText(e.getActionCommand() + " MenuItem clicked."); } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AWTMenuDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AWTMenuDemoVerify the following output. (Click on File Menu. Select any menu item.)
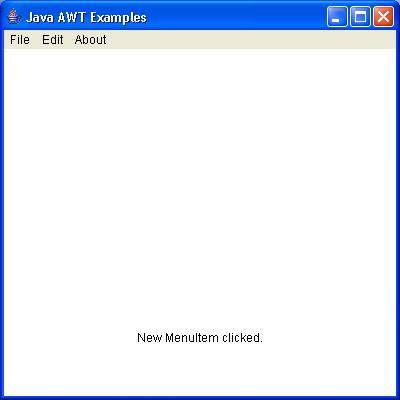
AWT Menu Class
Introduction
The Menu class represents pull-down menu component which is deployed from a menu bar.Class declaration
Following is the declaration for java.awt.Menu class:public class Menu extends MenuItem implements MenuContainer, Accessible
Class constructors
S.N. | Constructor & Description |
---|---|
1 | Menu() Constructs a new menu with an empty label. |
2 | Menu(String label) Constructs a new menu with the specified label. |
3 | Menu(String label, boolean tearOff) Constructs a new menu with the specified label, indicating whether the menu can be torn off. |
Class methods
S.N. | Method & Description |
---|---|
1 | MenuItem add(MenuItem mi) Adds the specified menu item to this menu. |
2 | void add(String label) Adds an item with the specified label to this menu. |
3 | void addNotify() Creates the menu's peer. |
4 | void addSeparator() Adds a separator line, or a hypen, to the menu at the current position. |
5 | int countItems() Deprecated. As of JDK version 1.1, replaced by getItemCount(). |
6 | AccessibleContext getAccessibleContext() Gets the AccessibleContext associated with this Menu. |
7 | MenuItem getItem(int index) Gets the item located at the specified index of this menu. |
8 | int getItemCount() Get the number of items in this menu. |
9 | void insert(MenuItem menuitem, int index) Inserts a menu item into this menu at the specified position. |
10 | void insert(String label, int index) Inserts a menu item with the specified label into this menu at the specified position. |
11 | void insertSeparator(int index) Inserts a separator at the specified position. |
12 | boolean isTearOff() Indicates whether this menu is a tear-off menu. |
13 | String paramString() Returns a string representing the state of this Menu. |
14 | void remove(int index) Removes the menu item at the specified index from this menu. |
15 | void remove(MenuComponent item) Removes the specified menu item from this menu. |
16 | void removeAll() Removes all items from this menu. |
17 | void removeNotify() Removes the menu's peer. |
Methods inherited
This class inherits methods from the following classes:- java.awt.MenuItem
- java.awt.MenuComponent
- java.lang.Object
Menu Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AWTMenuDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AWTMenuDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AWTMenuDemo(){ prepareGUI(); } public static void main(String[] args){ AWTMenuDemo awtMenuDemo = new AWTMenuDemo(); awtMenuDemo.showMenuDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showMenuDemo(){ //create a menu bar final MenuBar menuBar = new MenuBar(); //create menus Menu fileMenu = new Menu("File"); Menu editMenu = new Menu("Edit"); final Menu aboutMenu = new Menu("About"); //create menu items MenuItem newMenuItem = new MenuItem("New",new MenuShortcut(KeyEvent.VK_N)); newMenuItem.setActionCommand("New"); MenuItem openMenuItem = new MenuItem("Open"); openMenuItem.setActionCommand("Open"); MenuItem saveMenuItem = new MenuItem("Save"); saveMenuItem.setActionCommand("Save"); MenuItem exitMenuItem = new MenuItem("Exit"); exitMenuItem.setActionCommand("Exit"); MenuItem cutMenuItem = new MenuItem("Cut"); cutMenuItem.setActionCommand("Cut"); MenuItem copyMenuItem = new MenuItem("Copy"); copyMenuItem.setActionCommand("Copy"); MenuItem pasteMenuItem = new MenuItem("Paste"); pasteMenuItem.setActionCommand("Paste"); MenuItemListener menuItemListener = new MenuItemListener(); newMenuItem.addActionListener(menuItemListener); openMenuItem.addActionListener(menuItemListener); saveMenuItem.addActionListener(menuItemListener); exitMenuItem.addActionListener(menuItemListener); cutMenuItem.addActionListener(menuItemListener); copyMenuItem.addActionListener(menuItemListener); pasteMenuItem.addActionListener(menuItemListener); final CheckboxMenuItem showWindowMenu = new CheckboxMenuItem("Show About", true); showWindowMenu.addItemListener(new ItemListener() { public void itemStateChanged(ItemEvent e) { if(showWindowMenu.getState()){ menuBar.add(aboutMenu); }else{ menuBar.remove(aboutMenu); } } }); //add menu items to menus fileMenu.add(newMenuItem); fileMenu.add(openMenuItem); fileMenu.add(saveMenuItem); fileMenu.addSeparator(); fileMenu.add(showWindowMenu); fileMenu.addSeparator(); fileMenu.add(exitMenuItem); editMenu.add(cutMenuItem); editMenu.add(copyMenuItem); editMenu.add(pasteMenuItem); //add menu to menubar menuBar.add(fileMenu); menuBar.add(editMenu); menuBar.add(aboutMenu); //add menubar to the frame mainFrame.setMenuBar(menuBar); mainFrame.setVisible(true); } class MenuItemListener implements ActionListener { public void actionPerformed(ActionEvent e) { statusLabel.setText(e.getActionCommand() + " MenuItem clicked."); } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AWTMenuDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AWTMenuDemoVerify the following output. (Click on File Menu.)
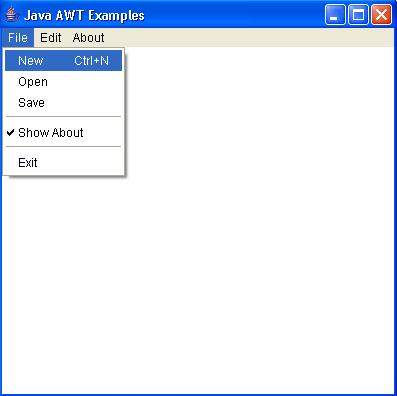
AWT CheckboxMenuItem Class
Introduction
The CheckboxMenuItem class represents a check box which can be included in a menu. Selecting the check box in the menu changes control's state from on to off or from off to on.Class declaration
Following is the declaration for java.awt.CheckboxMenuItem class:public class CheckboxMenuItem extends MenuItem implements ItemSelectable, Accessible
Class constructors
S.N. | Constructor & Description |
---|---|
1 | CheckboxMenuItem() Create a check box menu item with an empty label. |
2 | CheckboxMenuItem(String label) Create a check box menu item with the specified label. |
3 | CheckboxMenuItem(String label, boolean state) Create a check box menu item with the specified label and state. |
Class methods
S.N. | Method & Description |
---|---|
1 | void addItemListener(ItemListener l) Adds the specified item listener to receive item events from this check box menu item. |
2 | void addNotify() Creates the peer of the checkbox item. |
3 | AccessibleContext getAccessibleContext() Gets the AccessibleContext associated with this CheckboxMenuItem. |
4 | ItemListener[] getItemListeners() Returns an array of all the item listeners registered on this checkbox menuitem. |
5 | <T extends EventListener> T[] getListeners(Class<T> listenerType) Returns an array of all the objects currently registered as FooListeners upon this CheckboxMenuItem. |
6 | Object[] getSelectedObjects() Returns the an array (length 1) containing the checkbox menu item label or null if the checkbox is not selected. |
7 | boolean getState() Determines whether the state of this check box menu item is "on" or "off." |
8 | String paramString() Returns a string representing the state of this CheckBoxMenuItem. |
9 | protected void processEvent(AWTEvent e) Processes events on this check box menu item. |
10 | protected void processItemEvent(ItemEvent e) Processes item events occurring on this check box menu item by dispatching them to any registered ItemListener objects. |
11 | void removeItemListener(ItemListener l) Removes the specified item listener so that it no longer receives item events from this check box menu item. |
12 | void setState(boolean b) Sets this check box menu item to the specifed state. |
Methods inherited
This class inherits methods from the following classes:- java.awt.MenuItem
- java.awt.MenuComponent
- java.lang.Object
CheckboxMenuItem Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AWTMenuDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AWTMenuDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AWTMenuDemo(){ prepareGUI(); } public static void main(String[] args){ AWTMenuDemo awtMenuDemo = new AWTMenuDemo(); awtMenuDemo.showMenuDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showMenuDemo(){ //create a menu bar final MenuBar menuBar = new MenuBar(); //create menus Menu fileMenu = new Menu("File"); Menu editMenu = new Menu("Edit"); final Menu aboutMenu = new Menu("About"); //create menu items MenuItem newMenuItem = new MenuItem("New",new MenuShortcut(KeyEvent.VK_N)); newMenuItem.setActionCommand("New"); MenuItem openMenuItem = new MenuItem("Open"); openMenuItem.setActionCommand("Open"); MenuItem saveMenuItem = new MenuItem("Save"); saveMenuItem.setActionCommand("Save"); MenuItem exitMenuItem = new MenuItem("Exit"); exitMenuItem.setActionCommand("Exit"); MenuItem cutMenuItem = new MenuItem("Cut"); cutMenuItem.setActionCommand("Cut"); MenuItem copyMenuItem = new MenuItem("Copy"); copyMenuItem.setActionCommand("Copy"); MenuItem pasteMenuItem = new MenuItem("Paste"); pasteMenuItem.setActionCommand("Paste"); MenuItemListener menuItemListener = new MenuItemListener(); newMenuItem.addActionListener(menuItemListener); openMenuItem.addActionListener(menuItemListener); saveMenuItem.addActionListener(menuItemListener); exitMenuItem.addActionListener(menuItemListener); cutMenuItem.addActionListener(menuItemListener); copyMenuItem.addActionListener(menuItemListener); pasteMenuItem.addActionListener(menuItemListener); final CheckboxMenuItem showWindowMenu = new CheckboxMenuItem("Show About", true); showWindowMenu.addItemListener(new ItemListener() { public void itemStateChanged(ItemEvent e) { if(showWindowMenu.getState()){ menuBar.add(aboutMenu); }else{ menuBar.remove(aboutMenu); } } }); //add menu items to menus fileMenu.add(newMenuItem); fileMenu.add(openMenuItem); fileMenu.add(saveMenuItem); fileMenu.addSeparator(); fileMenu.add(showWindowMenu); fileMenu.addSeparator(); fileMenu.add(exitMenuItem); editMenu.add(cutMenuItem); editMenu.add(copyMenuItem); editMenu.add(pasteMenuItem); //add menu to menubar menuBar.add(fileMenu); menuBar.add(editMenu); menuBar.add(aboutMenu); //add menubar to the frame mainFrame.setMenuBar(menuBar); mainFrame.setVisible(true); } class MenuItemListener implements ActionListener { public void actionPerformed(ActionEvent e) { statusLabel.setText(e.getActionCommand() + " MenuItem clicked."); } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AWTMenuDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AWTMenuDemoVerify the following output. (Click on File Menu. Unselect "Show About" menu item.)
AWT PopupMenu Class
Introduction
Popup menu represents a menu which can be dynamically popped up at a specified position within a component.Class declaration
Following is the declaration for java.awt.PopupMenu class:public class CheckboxMenuItem extends MenuItem implements ItemSelectable, Accessible
Class constructors
S.N. | Constructor & Description |
---|---|
1 | PopupMenu() Creates a new popup menu with an empty name. |
2 | PopupMenu(String label) Creates a new popup menu with the specified name. |
Class methods
S.N. | Method & Description |
---|---|
1 | void addNotify() Creates the popup menu's peer. |
2 | AccessibleContext getAccessibleContext() Gets the AccessibleContext associated with this PopupMenu. |
3 | MenuContainer getParent() Returns the parent container for this menu component. |
4 | void show(Component origin, int x, int y) Shows the popup menu at the x, y position relative to an origin component. |
Methods inherited
This class inherits methods from the following classes:- java.awt.MenuItem
- java.awt.MenuComponent
- java.lang.Object
PopupMenu Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AWTMenuDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AWTMenuDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AWTMenuDemo(){ prepareGUI(); } public static void main(String[] args){ AWTMenuDemo awtMenuDemo = new AWTMenuDemo(); awtMenuDemo.showPopupMenuDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showPopupMenuDemo(){ final PopupMenu editMenu = new PopupMenu("Edit"); MenuItem cutMenuItem = new MenuItem("Cut"); cutMenuItem.setActionCommand("Cut"); MenuItem copyMenuItem = new MenuItem("Copy"); copyMenuItem.setActionCommand("Copy"); MenuItem pasteMenuItem = new MenuItem("Paste"); pasteMenuItem.setActionCommand("Paste"); MenuItemListener menuItemListener = new MenuItemListener(); cutMenuItem.addActionListener(menuItemListener); copyMenuItem.addActionListener(menuItemListener); pasteMenuItem.addActionListener(menuItemListener); editMenu.add(cutMenuItem); editMenu.add(copyMenuItem); editMenu.add(pasteMenuItem); controlPanel.addMouseListener(new MouseAdapter() { public void mouseClicked(MouseEvent e) { editMenu.show(controlPanel, e.getX(), e.getY()); } }); controlPanel.add(editMenu); mainFrame.setVisible(true); } class MenuItemListener implements ActionListener { public void actionPerformed(ActionEvent e) { statusLabel.setText(e.getActionCommand() + " MenuItem clicked."); } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AWTMenuDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AWTMenuDemoVerify the following output. (Click in the middle on the screen.)
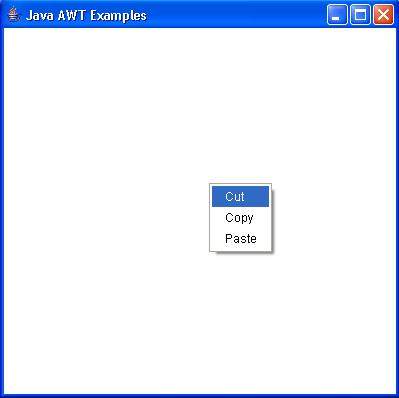
AWT Graphics Classes
Graphics controls allows application to draw onto the component or on image.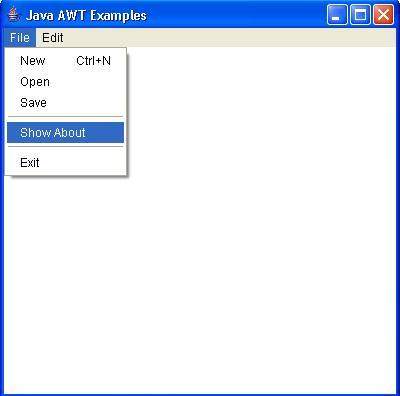
No comments:
Post a Comment