AWT MouseEvent Class
This event indicates a mouse action occurred in a component. This low-level event is generated by a component object for Mouse Events and Mouse motion events.- a mouse button is pressed
- a mouse button is released
- a mouse button is clicked (pressed and released)
- a mouse cursor enters the unobscured part of component's geometry
- a mouse cursor exits the unobscured part of component's geometry
- a mouse is moved
- the mouse is dragged
Class declaration
Following is the declaration for java.awt.event.MouseEvent class:public class MouseEvent extends InputEvent
Field
Following are the fields for java.awt.event.MouseEvent class:- static int BUTTON1 --Indicates mouse button #1; used by getButton()
- static int BUTTON2 --Indicates mouse button #2; used by getButton()
- static int BUTTON3 --Indicates mouse button #3; used by getButton()
- static int MOUSE_CLICKED --The "mouse clicked" event
- static int MOUSE_DRAGGED --The "mouse dragged" event
- static int MOUSE_ENTERED --The "mouse entered" event
- static int MOUSE_EXITED --The "mouse exited" event
- static int MOUSE_FIRST --The first number in the range of ids used for mouse events
- static int MOUSE_LAST -- The last number in the range of ids used for mouse events
- static int MOUSE_MOVED --The "mouse moved" event
- static int MOUSE_PRESSED -- The "mouse pressed" event
- static int MOUSE_RELEASED --The "mouse released" event
- static int MOUSE_WHEEL --The "mouse wheel" event
- static int NOBUTTON --Indicates no mouse buttons; used by getButton()
- static int VK_WINDOWS --Constant for the Microsoft Windows "Windows" key.
Class constructors
S.N. | Constructor & Description |
---|---|
1 | MouseEvent(Component source, int id, long when, int modifiers, int x, int y, int clickCount, boolean popupTrigger) Constructs a MouseEvent object with the specified source component, type, modifiers, coordinates, and click count. |
2 | MouseEvent(Component source, int id, long when, int modifiers, int x, int y, int clickCount, boolean popupTrigger, int button) Constructs a MouseEvent object with the specified source component, type, modifiers, coordinates, and click count. |
3 | MouseEvent(Component source, int id, long when, int
modifiers, int x, int y, int xAbs, int yAbs, int clickCount, boolean
popupTrigger, int button) Constructs a MouseEvent object with the specified source component, type, modifiers, coordinates, absolute coordinates, and click count. |
Class methods
S.N. | Method & Description |
---|---|
1 | int getButton() Returns which, if any, of the mouse buttons has changed state. |
2 | int getClickCount() Returns the number of mouse clicks associated with this event. |
3 | Point getLocationOnScreen() Returns the absolute x, y position of the event. |
4 | static String getMouseModifiersText(int modifiers) Returns a String describing the modifier keys and mouse buttons that were down during the event, such as "Shift", or "Ctrl+Shift". |
5 | Point getPoint() Returns the x,y position of the event relative to the source component. |
6 | int getX() Returns the horizontal x position of the event relative to the source component. |
7 | int getXOnScreen() Returns the absolute horizontal x position of the event. |
8 | int getY() Returns the vertical y position of the event relative to the source component. |
9 | int getYOnScreen() Returns the absolute vertical y position of the event. |
10 | boolean isPopupTrigger() Returns whether or not this mouse event is the popup menu trigger event for the platform. |
11 | String paramString() Returns a parameter string identifying this event. |
12 | void translatePoint(int x, int y) Translates the event's coordinates to a new position by adding specified x (horizontal) and y (vertical) offsets. |
Methods inherited
This class inherits methods from the following classes:- java.awt.event.InputEvent
- java.awt.event.ComponentEvent
- java.awt.AWTEvent
- java.util.EventObject
- java.lang.Object
AWT TextEvent Class
The object of this class represents the text events.The TextEvent is generated when character is entered in the text fields or text area. The TextEvent instance does not include the characters currently in the text component that generated the event rather we are provided with other methods to retrieve that information.Class declaration
Following is the declaration for java.awt.event.TextEvent class:public class TextEvent extends AWTEvent
Field
Following are the fields for java.awt.event.TextEvent class:- static int TEXT_FIRST --The first number in the range of ids used for text events.
- static int TEXT_LAST --The last number in the range of ids used for text events.
- static int TEXT_VALUE_CHANGED --This event id indicates that object's text changed.
Class constructors
<0tr>S.N. | Constructor & Description |
---|---|
1 | TextEvent(Object source, int id) Constructs a TextEvent object. |
Class methods
S.N. | Method & Description |
---|---|
1 | String paramString() Returns a parameter string identifying this text event. |
Methods inherited
This class inherits methods from the following classes:- java.awt.AWTEvent
- java.util.EventObject
- java.lang.Object
AWT WindowEvent Class
The object of this class represents the change in state of a window.This low-level event is generated by a Window object when it is opened, closed, activated, deactivated, iconified, or deiconified, or when focus is transfered into or out of the Window.Class declaration
Following is the declaration for java.awt.event.WindowEvent class:public class WindowEvent extends ComponentEvent
Field
Following are the fields for java.awt.event.WindowEvent class:- static int WINDOW_ACTIVATED --The window-activated event type.
- static int WINDOW_CLOSED -- The window closed event.
- static int WINDOW_CLOSING -- The "window is closing" event.
- static int WINDOW_DEACTIVATED -- The window-deactivated event type.
- static int WINDOW_DEICONIFIED -- The window deiconified event type.
- static int WINDOW_FIRST -- The first number in the range of ids used for window events.
- static int WINDOW_GAINED_FOCUS -- The window-gained-focus event type.
- static int WINDOW_ICONIFIED -- The window iconified event.
- static int WINDOW_LAST -- The last number in the range of ids used for window events.
- static int WINDOW_LOST_FOCUS -- The window-lost-focus event type.
- static int WINDOW_OPENED -- The window opened event.
- static int WINDOW_STATE_CHANGED -- The window-state-changed event type.
Class constructors
<0tr>S.N. | Constructor & Description |
---|---|
1 | WindowEvent(Window source, int id) Constructs a WindowEvent object. |
2 | WindowEvent(Window source, int id, int oldState, int newState) Constructs a WindowEvent object with the specified previous and new window states. |
3 | WindowEvent(Window source, int id, Window opposite) Constructs a WindowEvent object with the specified opposite Window. |
4 | WindowEvent(Window source, int id, Window opposite, int oldState, int newState) Constructs a WindowEvent object. |
Class methods
S.N. | Method & Description |
---|---|
1 | int getNewState() For WINDOW_STATE_CHANGED events returns the new state of the window. |
2 | int getOldState() For WINDOW_STATE_CHANGED events returns the previous state of the window. |
3 | Window getOppositeWindow() Returns the other Window involved in this focus or activation change. |
4 | Window getWindow() Returns the originator of the event. |
5 | String paramString() Returns a parameter string identifying this event. |
Methods inherited
This class inherits methods from the following classes:- java.awt.event.ComponentEvent
- java.awt.AWTEvent
- java.util.EventObject
- java.lang.Object
AWT AdjustmentEvent Class
Introduction
The Class AdjustmentEvent represents adjustment event emitted by Adjustable objects.Class declaration
Following is the declaration for java.awt.event.AdjustmentEvent class:public class AdjustmentEvent extends AWTEvent
Field
Following are the fields for java.awt.Component class:- static int ADJUSTMENT_FIRST -- Marks the first integer id for the range of adjustment event ids.
- static int ADJUSTMENT_LAST -- Marks the last integer id for the range of adjustment event ids.
- static int ADJUSTMENT_VALUE_CHANGED -- The adjustment value changed event.
- static int BLOCK_DECREMENT -- The block decrement adjustment type.
- static int BLOCK_INCREMENT -- The block increment adjustment type.
- static int TRACK -- The absolute tracking adjustment type.
- static int UNIT_DECREMENT -- The unit decrement adjustment type.
- static int UNIT_INCREMENT -- The unit increment adjustment type.
Class constructors
S.N. | Constructor & Description |
---|---|
1 | AdjustmentEvent(Adjustable source, int id, int type, int value) Constructs an AdjustmentEvent object with the specified Adjustable source, event type, adjustment type, and value. |
2 | AdjustmentEvent(Adjustable source, int id, int type, int value, boolean isAdjusting) Constructs an AdjustmentEvent object with the specified Adjustable source, event type, adjustment type, and value. |
Class methods
S.N. | Method & Description |
---|---|
1 | Adjustable getAdjustable() Returns the Adjustable object where this event originated. |
2 | int getAdjustmentType() Returns the type of adjustment which caused the value changed event. |
3 | int getValue() Returns the current value in the adjustment event. |
4 | boolean getValueIsAdjusting() Returns true if this is one of multiple adjustment events. |
5 | String paramString() Returns a string representing the state of this Event. |
Methods inherited
This interface inherits methods from the following classes:- java.awt.AWTEvent
- java.util.EventObject
- java.lang.Object
AWT ComponentEvent Class
Introduction
The Class ComponentEvent represents that a component moved, changed size, or changed visibilityClass declaration
Following is the declaration for java.awt.event.ComponentEvent class:public class ComponentEvent extends AWTEvent
Field
Following are the fields for java.awt.Component class:- static int COMPONENT_FIRST -- The first number in the range of ids used for component events.
- static int COMPONENT_HIDDEN --This event indicates that the component was rendered invisible.
- static int COMPONENT_LAST -- The last number in the range of ids used for component events.
- static int COMPONENT_MOVED -- This event indicates that the component's position changed.
- static int COMPONENT_RESIZED -- This event indicates that the component's size changed.
- static int COMPONENT_SHOWN -- This event indicates that the component was made visible.
Class constructors
S.N. | Constructor & Description |
---|---|
1 | ComponentEvent(Component source, int id) Constructs a ComponentEvent object. |
Class methods
S.N. | Method & Description |
---|---|
1 | Component getComponent() Returns the originator of the event. |
2 | String paramString() Returns a parameter string identifying this event. |
Methods inherited
This interface inherits methods from the following classes:- java.awt.AWTEvent
- java.util.EventObject
- java.lang.Object
AWT ContainerEvent Class
Introduction
The Class ContainerEvent represents that a container's contents changed because a component was added or removed.Class declaration
Following is the declaration for java.awt.event.ContainerEvent class:public class ContainerEvent extends ComponentEvent
Field
Following are the fields for java.awt.Component class:- static int COMPONENT_ADDED -- This event indicates that a component was added to the container.
- static int COMPONENT_REMOVED -- This event indicates that a component was removed from the container.
- static int CONTAINER_FIRST -- The first number in the range of ids used for container events.
- static int CONTAINER_LAST -- The last number in the range of ids used for container events.
Class constructors
Class methods
S.N. | Constructor & Description |
---|---|
1 | ContainerEvent(Component source, int id, Component child) Constructs a ContainerEvent object. |
S.N. | Method & Description |
---|---|
1 | Component getChild() Returns the component that was affected by the event. |
2 | Container getContainer() Returns the originator of the event. |
3 | String paramString() Returns a parameter string identifying this event. |
Methods inherited
This class inherits methods from the following classes:- java.awt.ComponentEvent
- java.awt.AWTEvent
- java.util.EventObject
- java.lang.Object
AWT MouseMotionEvent Class
Introduction
The interface MouseMotionEvent indicates a mouse action occurred in a component. This low-level event is generated by a component object when mouse is dragged or moved.Class declaration
Following is the declaration for java.awt.event.MouseMotionEvent Class:public class MouseMotionEvent extends InputEvent
Interface methods
S.N. | Method & Description |
---|---|
1 | void mouseDragged(MouseEvent e) Invoked when a mouse button is pressed on a component and then dragged. |
2 | void mouseMoved(MouseEvent e) Invoked when the mouse cursor has been moved onto a component but no buttons have been pushed. |
Methods inherited
This interface inherits methods from the following classes:- java.awt.event.InputEvent
- java.awt.event.ComponentEvent
- java.awt.AWTEvent
- java.util.EventObject
- java.lang.Object
AWT PaintEvent Class
Introduction
The Class PaintEvent used to ensure that paint/update method calls are serialized along with the other events delivered from the event queueClass declaration
Following is the declaration for java.awt.event.PaintEvent class:public class PaintEvent extends ComponentEvent
Field
Following are the fields for java.awt.Component class:- static int PAINT -- The paint event type.
- static int PAINT_FIRST -- Marks the first integer id for the range of paint event ids.
- static int PAINT_LAST -- Marks the last integer id for the range of paint event ids.
- static int UPDATE -- The update event type.
Class constructors
S.N. | Constructor & Description |
---|---|
1 | PaintEvent(Component source, int id, Rectangle updateRect) Constructs a PaintEvent object with the specified source component and type. |
Class methods
S.N. | Method & Description |
---|---|
1 | Rectangle getUpdateRect() Returns the rectangle representing the area which needs to be repainted in response to this event. |
2 | String paramString() Returns a parameter string identifying this event. |
3 | void setUpdateRect(Rectangle updateRect) Sets the rectangle representing the area which needs to be repainted in response to this event. |
Methods inherited
This class inherits methods from the following classes:- java.awt.ComponentEvent
- java.awt.AWTEvent
- java.util.EventObject
- java.lang.Object
AWT Event Listeners
The Event listener represent the interfaces responsible to handle events. Java provides us various Event listener classes but we will discuss those which are more frequently used. Every method of an event listener method has a single argument as an object which is subclass of EventObject class. For example, mouse event listener methods will accept instance of MouseEvent, where MouseEvent derives from EventObject.EventListner interface
It is a marker interface which every listener interface has to extend.This class is defined in java.util package.Class declaration
Following is the declaration for java.util.EventListener interface:public interface EventListener
AWT Event Listener Interfaces:
Following is the list of commonly used event listeners.AWT ActionListener Interface
The class which processes the ActionEvent should implement this interface.The object of that class must be registered with a component. The object can be registered using the addActionListener() method. When the action event occurs, that object's actionPerformed method is invoked.Interface declaration
Following is the declaration for java.awt.event.ActionListener interface:public interface ActionListener extends EventListener
Interface methods
S.N. | Method & Description |
---|---|
1 | void actionPerformed(ActionEvent e) Invoked when an action occurs. |
Methods inherited
This interface inherits methods from the following interfaces:- java.awt.EventListener
ActionListener Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtListenerDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtListenerDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtListenerDemo(){ prepareGUI(); } public static void main(String[] args){ AwtListenerDemo awtListenerDemo = new AwtListenerDemo(); awtListenerDemo.showActionListenerDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showActionListenerDemo(){ headerLabel.setText("Listener in action: ActionListener"); ScrollPane panel = new ScrollPane(); panel.setBackground(Color.magenta); Button okButton = new Button("OK"); okButton.addActionListener(new CustomActionListener()); panel.add(okButton); controlPanel.add(panel); mainFrame.setVisible(true); } class CustomActionListener implements ActionListener{ public void actionPerformed(ActionEvent e) { statusLabel.setText("Ok Button Clicked."); } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtListenerDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtListenerDemoVerify the following output
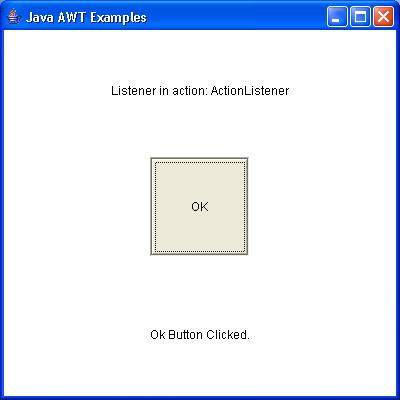
AWT ComponentListener Interface
The class which processes the ComponentEvent should implement this interface.The object of that class must be registered with a component. The object can be registered using the addComponentListener() method. Component event are raised for information only.Interface declaration
Following is the declaration for java.awt.event.ComponentListener interface:public interface ComponentListener extends EventListener
Interface methods
S.N. | Method & Description |
---|---|
1 | void componentHidden(ComponentEvent e) Invoked when the component has been made invisible. |
2 | void componentMoved(ComponentEvent e) Invoked when the component's position changes. |
3 | void componentResized(ComponentEvent e) Invoked when the component's size changes. |
4 | void componentShown(ComponentEvent e) Invoked when the component has been made visible. |
Methods inherited
This interface inherits methods from the following interfaces:- java.awt.EventListener
ComponentListener Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtListenerDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtListenerDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtListenerDemo(){ prepareGUI(); } public static void main(String[] args){ AwtListenerDemo awtListenerDemo = new AwtListenerDemo(); awtListenerDemo.showComponentListenerDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showComponentListenerDemo(){ headerLabel.setText("Listener in action: ComponentListener"); ScrollPane panel = new ScrollPane(); panel.setBackground(Color.magenta); Label msglabel = new Label(); msglabel.setAlignment(Label.CENTER); msglabel.setText("Welcome to TutorialsPoint AWT Tutorial."); panel.add(msglabel); msglabel.addComponentListener(new CustomComponentListener()); controlPanel.add(panel); mainFrame.setVisible(true); } class CustomComponentListener implements ComponentListener { public void componentResized(ComponentEvent e) { statusLabel.setText(statusLabel.getText() + e.getComponent().getClass().getSimpleName() + " resized. "); } public void componentMoved(ComponentEvent e) { statusLabel.setText(statusLabel.getText() + e.getComponent().getClass().getSimpleName() + " moved. "); } public void componentShown(ComponentEvent e) { statusLabel.setText(statusLabel.getText() + e.getComponent().getClass().getSimpleName() + " shown. "); } public void componentHidden(ComponentEvent e) { statusLabel.setText(statusLabel.getText() + e.getComponent().getClass().getSimpleName() + " hidden. "); } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtListenerDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtListenerDemoVerify the following output
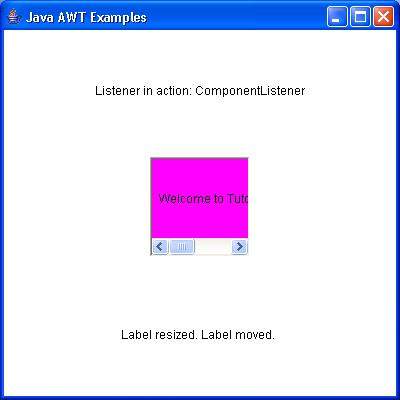
AWT ItemListener Interface
The class which processes the ItemEvent should implement this interface.The object of that class must be registered with a component. The object can be registered using the addItemListener() method. When the action event occurs, that object's itemStateChanged method is invoked.Interface declaration
Following is the declaration for java.awt.event.ItemListener interface:public interface ItemListener extends EventListener
Interface methods
S.N. | Method & Description |
---|---|
1 | void itemStateChanged(ItemEvent e) Invoked when an item has been selected or deselected by the user. |
Methods inherited
This interface inherits methods from the following interfaces:- java.awt.EventListener
ItemListener Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtListenerDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtListenerDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtListenerDemo(){ prepareGUI(); } public static void main(String[] args){ AwtListenerDemo awtListenerDemo = new AwtListenerDemo(); awtListenerDemo.showItemListenerDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showItemListenerDemo(){ headerLabel.setText("Listener in action: ItemListener"); Checkbox chkApple = new Checkbox("Apple"); Checkbox chkMango = new Checkbox("Mango"); Checkbox chkPeer = new Checkbox("Peer"); chkApple.addItemListener(new CustomItemListener()); chkMango.addItemListener(new CustomItemListener()); chkPeer.addItemListener(new CustomItemListener()); controlPanel.add(chkApple); controlPanel.add(chkMango); controlPanel.add(chkPeer); mainFrame.setVisible(true); } class CustomItemListener implements ItemListener { public void itemStateChanged(ItemEvent e) { statusLabel.setText(e.getItem() +" Checkbox: " + (e.getStateChange()==1?"checked":"unchecked")); } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtListenerDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtListenerDemoVerify the following output
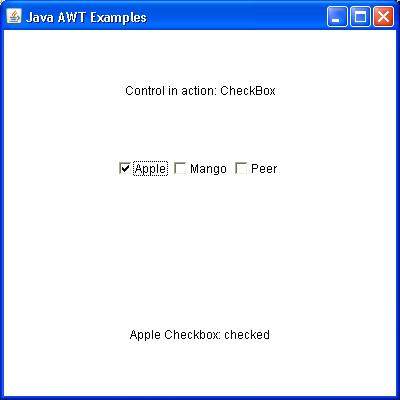
AWT KeyListener Interface
The class which processes the KeyEvent should implement this interface.The object of that class must be registered with a component. The object can be registered using the addKeyListener() method.Interface declaration
Following is the declaration for java.awt.event.KeyListener interface:public interface KeyListener extends EventListener
Interface methods
S.N. | Method & Description |
---|---|
1 | void keyPressed(KeyEvent e) Invoked when a key has been pressed. |
2 | void keyReleased(KeyEvent e) Invoked when a key has been released. |
3 | void keyTyped(KeyEvent e) Invoked when a key has been typed. |
Methods inherited
This interface inherits methods from the following interfaces:- java.awt.EventListener
KeyListener Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtListenerDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtListenerDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; private TextField textField; public AwtListenerDemo(){ prepareGUI(); } public static void main(String[] args){ AwtListenerDemo awtListenerDemo = new AwtListenerDemo(); awtListenerDemo.showKeyListenerDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showKeyListenerDemo(){ headerLabel.setText("Listener in action: KeyListener"); textField = new TextField(10); textField.addKeyListener(new CustomKeyListener()); Button okButton = new Button("OK"); okButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { statusLabel.setText("Entered text: " + textField.getText()); } }); controlPanel.add(textField); controlPanel.add(okButton); mainFrame.setVisible(true); } class CustomKeyListener implements KeyListener{ public void keyTyped(KeyEvent e) { } public void keyPressed(KeyEvent e) { if(e.getKeyCode() == KeyEvent.VK_ENTER){ statusLabel.setText("Entered text: " + textField.getText()); } } public void keyReleased(KeyEvent e) { } } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtListenerDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtListenerDemoVerify the following output
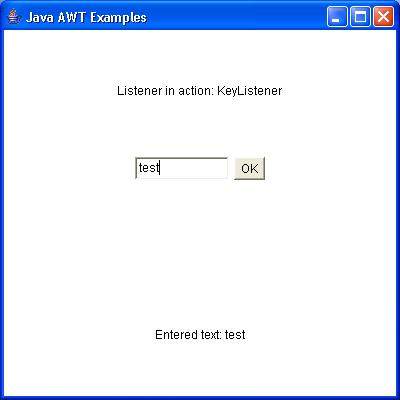
No comments:
Post a Comment