AWT Rectangle2D Class
Introduction
The Rectangle2D class states a rectangle defined by a location (x,y) and dimension (w x h).Class declaration
Following is the declaration for java.awt.geom.Rectangle2D class:public abstract class Rectangle2D extends RectangularShape
Field
Following are the fields for java.awt.geom.Arc2D class:- static int OUT_BOTTOM -- The bitmask that indicates that a point lies below this Rectangle2D.
- static int OUT_LEFT -- The bitmask that indicates that a point lies to the left of this Rectangle2D.
- static int OUT_RIGHT -- The bitmask that indicates that a point lies to the right of this Rectangle2D.
- static int OUT_TOP -- The bitmask that indicates that a point lies above this Rectangle2D.
Class constructors
S.N. | Constructor & Description |
---|---|
1 | protected Rectangle2D() This is an abstract class that cannot be instantiated directly. |
Class methods
S.N. | Method & Description |
---|---|
1 | void add(double newx, double newy) Adds a point, specified by the double precision arguments newx and newy, to this Rectangle2D. |
2 | void add(Point2D pt) Adds the Point2D object pt to this Rectangle2D. |
3 | void add(Rectangle2D r) Adds a Rectangle2D object to this Rectangle2D. |
4 | boolean contains(double x, double y) Tests if the specified coordinates are inside the boundary of the Shape. |
5 | boolean contains(double x, double y, double w, double h) Tests if the interior of the Shape entirely contains the specified rectangular area. |
6 | abstract Rectangle2D createIntersection(Rectangle2D r) Returns a new Rectangle2D object representing the intersection of this Rectangle2D with the specified Rectangle2D. |
7 | abstract Rectangle2D createUnion(Rectangle2D r) Returns a new Rectangle2D object representing the union of this Rectangle2D with the specified Rectangle2D. |
8 | boolean equals(Object obj) Determines whether or not the specified Object is equal to this Rectangle2D. |
9 | Rectangle2D getBounds2D() Returns a high precision and more accurate bounding box of the Shape than the getBounds method. |
10 | PathIterator getPathIterator(AffineTransform at) Returns an iteration object that defines the boundary of this Rectangle2D. |
11 | PathIterator getPathIterator(AffineTransform at, double flatness) Returns an iteration object that defines the boundary of the flattened Rectangle2D. |
12 | int hashCode() Returns the hashcode for this Rectangle2D. |
13 | static void intersect(Rectangle2D src1, Rectangle2D src2, Rectangle2D dest) Intersects the pair of specified source Rectangle2D objects and puts the result into the specified destination Rectangle2D object. |
14 | boolean intersects(double x, double y, double w, double h) Tests if the interior of the Shape intersects the interior of a specified rectangular area. |
15 | boolean intersectsLine(double x1, double y1, double x2, double y2) Tests if the specified line segment intersects the interior of this Rectangle2D. |
16 | boolean intersectsLine(Line2D l) Tests if the specified line segment intersects the interior of this Rectangle2D. |
17 | abstract int outcode(double x, double y) Determines where the specified coordinates lie with respect to this Rectangle2D. |
18 | int outcode(Point2D p) Determines where the specified Point2D lies with respect to this Rectangle2D. |
19 | void setFrame(double x, double y, double w, double h) Sets the location and size of the outer bounds of this Rectangle2D to the specified rectangular values. |
20 | abstract void setRect(double x, double y, double w, double h) Sets the location and size of this Rectangle2D to the specified double values. |
21 | void setRect(Rectangle2D r) Sets this Rectangle2D to be the same as the specified Rectangle2D. |
22 | static void union(Rectangle2D src1, Rectangle2D src2, Rectangle2D dest) Unions the pair of source Rectangle2D objects and puts the result into the specified destination Rectangle2D object. |
Methods inherited
This class inherits methods from the following classes:- java.awt.geom.RectangularShape
- java.lang.Object
Ellipse2D Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AWTGraphicsDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; import java.awt.geom.*; public class AWTGraphicsDemo extends Frame { public AWTGraphicsDemo(){ super("Java AWT Examples"); prepareGUI(); } public static void main(String[] args){ AWTGraphicsDemo awtGraphicsDemo = new AWTGraphicsDemo(); awtGraphicsDemo.setVisible(true); } private void prepareGUI(){ setSize(400,400); addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); } @Override public void paint(Graphics g) { Rectangle2D shape = new Rectangle2D.Float(); shape.setFrame(100, 150, 200,100); Graphics2D g2 = (Graphics2D) g; g2.draw (shape); Font font = new Font("Serif", Font.PLAIN, 24); g2.setFont(font); g.drawString("Welcome to TutorialsPoint", 50, 70); g2.drawString("Rectangle2D.Rectangle", 100, 120); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AWTGraphicsDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AWTGraphicsDemoVerify the following output
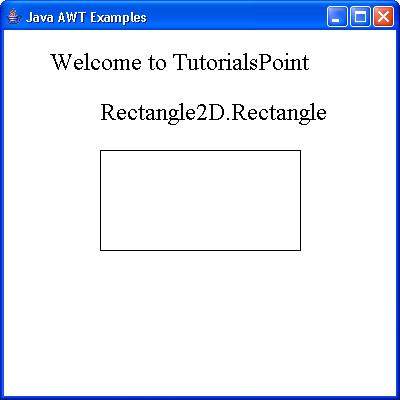
AWT QuadCurve2D Class
Introduction
The QuadCurve2D class states a quadratic parametric curve segment in (x,y) coordinate space.Class declaration
Following is the declaration for java.awt.geom.QuadCurve2D class:public abstract class QuadCurve2D extends Object implements Shape, Cloneable
Class constructors
S.N. | Constructor & Description |
---|---|
1 | protected QuadCurve2D() () This is an abstract class that cannot be instantiated directly. |
Class methods
S.N. | Method & Description |
---|---|
1 | Object clone() Creates a new object of the same class and with the same contents as this object. |
2 | boolean contains(double x, double y) Tests if the specified coordinates are inside the boundary of the Shape. |
3 | boolean contains(double x, double y, double w, double h) Tests if the interior of the Shape entirely contains the specified rectangular area. |
4 | boolean contains(Point2D p) Tests if a specified Point2D is inside the boundary of the Shape. |
5 | boolean contains(Rectangle2D r) Tests if the interior of the Shape entirely contains the specified Rectangle2D. |
6 | Rectangle getBounds() Returns an integer Rectangle that completely encloses the Shape. |
7 | abstract Point2D getCtrlPt() Returns the control point. |
8 | abstract double getCtrlX() Returns the X coordinate of the control point in double precision. |
9 | abstract double getCtrlY() Returns the Y coordinate of the control point in double precision. |
10 | doublegetFlatness() Returns the flatness, or maximum distance of a control point from the line connecting the end points, of this QuadCurve2D. |
11 | static double getFlatness(double[] coords, int offset) Returns the flatness, or maximum distance of a control point from the line connecting the end points, of the quadratic curve specified by the control points stored in the indicated array at the indicated index. |
12 | static double getFlatness(double x1, double y1, double ctrlx, double ctrly, double x2, double y2) Returns the flatness, or maximum distance of a control point from the line connecting the end points, of the quadratic curve specified by the indicated control points. |
13 | double getFlatnessSq() Returns the square of the flatness, or maximum distance of a control point from the line connecting the end points, of this QuadCurve2D. |
14 | static double getFlatnessSq(double[] coords, int offset) Returns the square of the flatness, or maximum distance of a control point from the line connecting the end points, of the quadratic curve specified by the control points stored in the indicated array at the indicated index. |
15 | static double getFlatnessSq(double x1, double y1, double ctrlx, double ctrly, double x2, double y2) Returns the square of the flatness, or maximum distance of a control point from the line connecting the end points, of the quadratic curve specified by the indicated control points. |
16 | abstract Point2D getP1() Returns the start point. |
17 | abstract Point2D getP2() Returns the end point. |
18 | PathIterator getPathIterator(AffineTransform at) Returns an iteration object that defines the boundary of the shape of this QuadCurve2D. |
19 | PathIterator getPathIterator(AffineTransform at, double flatness) Returns an iteration object that defines the boundary of the flattened shape of this QuadCurve2D. |
20 | abstract double getX1() Returns the X coordinate of the start point in double in precision. |
21 | abstract double getX2() Returns the X coordinate of the end point in double precision. |
22 | abstract double getY1() Returns the Y coordinate of the start point in double precision. |
23 | abstract double getY2() Returns the Y coordinate of the end point in double precision. |
24 | boolean intersects(double x, double y, double w, double h) Tests if the interior of the Shape intersects the interior of a specified rectangular area. |
25 | boolean intersects(Rectangle2D r) Tests if the interior of the Shape intersects the interior of a specified Rectangle2D. |
26 | void setCurve(double[] coords, int offset) Sets the location of the end points and control points of this QuadCurve2D to the double coordinates at the specified offset in the specified array. |
27 | abstract void setCurve(double x1, double y1, double ctrlx, double ctrly, double x2, double y2) Sets the location of the end points and control point of this curve to the specified double coordinates. |
28 | void setCurve(Point2D[] pts, int offset) Sets the location of the end points and control points of this QuadCurve2D to the coordinates of the Point2D objects at the specified offset in the specified array. |
29 | void setCurve(Point2D p1, Point2D cp, Point2D p2) Sets the location of the end points and control point of this QuadCurve2D to the specified Point2D coordinates. |
30 | void setCurve(QuadCurve2D c) Sets the location of the end points and control point of this QuadCurve2D to the same as those in the specified QuadCurve2D. |
31 | static int solveQuadratic(double[] eqn) Solves the quadratic whose coefficients are in the eqn array and places the non-complex roots back into the same array, returning the number of roots. |
32 | static int solveQuadratic(double[] eqn, double[] res) Solves the quadratic whose coefficients are in the eqn array and places the non-complex roots into the res array, returning the number of roots. |
33 | static void subdivide(double[] src, int srcoff, double[] left, int leftoff, double[] right, int rightoff) Subdivides the quadratic curve specified by the coordinates stored in the src array at indices srcoff through srcoff + 5 and stores the resulting two subdivided curves into the two result arrays at the corresponding indices. |
34 | void subdivide(QuadCurve2D left, QuadCurve2D right) Subdivides this QuadCurve2D and stores the resulting two subdivided curves into the left and right curve parameters. |
35 | static void subdivide(QuadCurve2D src, QuadCurve2D left, QuadCurve2D right) Subdivides the quadratic curve specified by the src parameter and stores the resulting two subdivided curves into the left and right curve parameters. |
Methods inherited
This class inherits methods from the following classes:- java.lang.Object
QuadCurve2D Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AWTGraphicsDemo
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; import java.awt.geom.*; public class AWTGraphicsDemo extends Frame { public AWTGraphicsDemo(){ super("Java AWT Examples"); prepareGUI(); } public static void main(String[] args){ AWTGraphicsDemo awtGraphicsDemo = new AWTGraphicsDemo(); awtGraphicsDemo.setVisible(true); } private void prepareGUI(){ setSize(400,400); addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); } @Override public void paint(Graphics g) { QuadCurve2D shape = new QuadCurve2D.Double(); shape.setCurve(250D,250D,100D,100D,200D,150D); Graphics2D g2 = (Graphics2D) g; g2.draw (shape); Font font = new Font("Serif", Font.PLAIN, 24); g2.setFont(font); g.drawString("Welcome to TutorialsPoint", 50, 70); g2.drawString("QuadCurve2D.Curve", 100, 120); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AWTGraphicsDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AWTGraphicsDemoVerify the following output
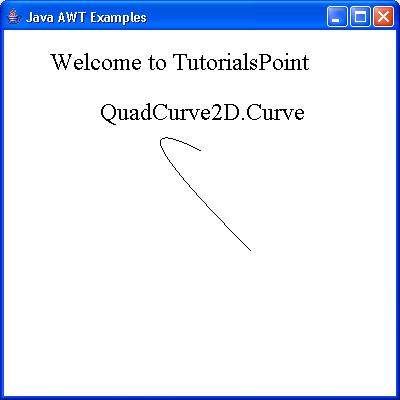
AWT Line2D Class
Introduction
The Line2D class states a line segment in (x,y) coordinate space.Class declaration
Following is the declaration for java.awt.geom.Line2D class:public abstract class Line2D extends Object implements Shape, Cloneable
Class constructors
S.N. | Constructor & Description |
---|---|
1 | protected Line2D() () This is an abstract class that cannot be instantiated directly. |
Class methods
S.N. | Method & Description |
---|---|
1 | Object clone() Creates a new object of the same class as this object. |
2 | boolean contains(double x, double y) Tests if a specified coordinate is inside the boundary of this Line2D. |
3 | boolean contains(double x, double y, double w, double h) Tests if the interior of this Line2D entirely contains the specified set of rectangular coordinates. |
4 | boolean contains(Point2D p) Tests if a given Point2D is inside the boundary of this Line2D. |
5 | boolean contains(Rectangle2D r) Tests if the interior of this Line2D entirely contains the specified Rectangle2D. |
6 | Rectangle getBounds() Returns an integer Rectangle that completely encloses the Shape. |
7 | abstract Point2D getP1() Returns the start Point2D of this Line2D. |
8 | abstract Point2D getP2() Returns the end Point2D of this Line2D. |
9 | PathIterator getPathIterator(AffineTransform at) Returns an iteration object that defines the boundary of this Line2D. |
10 | PathIterator getPathIterator(AffineTransform at, double flatness) Returns an iteration object that defines the boundary of this flattened Line2D. |
11 | abstract double getX1() Returns the X coordinate of the start point in double precision. |
12 | abstract double getX2() Returns the X coordinate of the end point in double precision. |
13 | abstract double getY1() Returns the Y coordinate of the start point in double precision. |
14 | abstract double getY2() Returns the Y coordinate of the end point in double precision. |
15 | boolean intersects(double x, double y, double w, double h) Tests if the interior of the Shape intersects the interior of a specified rectangular area. |
16 | boolean intersects(Rectangle2D r) Tests if the interior of the Shape intersects the interior of a specified Rectangle2D. |
17 | boolean intersectsLine(double x1, double y1, double x2, double y2) Tests if the line segment from (x1,y1) to (x2,y2) intersects this line segment. |
18 | boolean intersectsLine(Line2D l) Tests if the specified line segment intersects this line segment. |
19 | static boolean linesIntersect(double x1, double y1, double x2, double y2, double x3, double y3, double x4, double y4) Tests if the line segment from (x1,y1) to (x2,y2) intersects the line segment from (x3,y3) to (x4,y4). |
20 | double ptLineDist(double px, double py) Returns the distance from a point to this line. |
21 | static double ptLineDist(double x1, double y1, double x2, double y2, double px, double py) Returns the distance from a point to a line. |
22 | double ptLineDist(Point2D pt) Returns the distance from a Point2D to this line. |
23 | double ptLineDistSq(double px, double py) Returns the square of the distance from a point to this line. |
24 | static double ptLineDistSq(double x1, double y1, double x2, double y2, double px, double py) Returns the square of the distance from a point to a line. |
25 | double ptLineDistSq(Point2D pt) Returns the square of the distance from a specified Point2D to this line. |
26 | double ptSegDist(double px, double py) Returns the distance from a point to this line segment. |
27 | static double ptSegDist(double x1, double y1, double x2, double y2, double px, double py) Returns the distance from a point to a line segment. |
28 | double ptSegDist(Point2D pt) Returns the distance from a Point2D to this line segment. |
29 | double ptSegDistSq(double px, double py) Returns the square of the distance from a point to this line segment. |
30 | static double ptSegDistSq(double x1, double y1, double x2, double y2, double px, double py) Returns the square of the distance from a point to a line segment. |
31 | double ptSegDistSq(Point2D pt) Returns the square of the distance from a Point2D to this line segment. |
32 | int relativeCCW(double px, double py) Returns an indicator of where the specified point (px,py) lies with respect to this line segment. |
33 | static int relativeCCW(double x1, double y1, double x2, double y2, double px, double py) Returns an indicator of where the specified point (px,py) lies with respect to the line segment from (x1,y1) to (x2,y2). |
34 | int relativeCCW(Point2D p) Returns an indicator of where the specified Point2D lies with respect to this line segment. |
35 | abstract void setLine(double x1, double y1, double x2, double y2) Sets the location of the end points of this Line2D to the specified double coordinates. |
36 | void setLine(Line2D l) Sets the location of the end points of this Line2D to the same as those end points of the specified Line2D. |
37 | void setLine(Point2D p1, Point2D p2) Sets the location of the end points of this Line2D to the specified Point2D coordinates. |
Methods inherited
This class inherits methods from the following classes:- java.lang.Object
Line2D Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AWTGraphicsDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; import java.awt.geom.*; public class AWTGraphicsDemo extends Frame { public AWTGraphicsDemo(){ super("Java AWT Examples"); prepareGUI(); } public static void main(String[] args){ AWTGraphicsDemo awtGraphicsDemo = new AWTGraphicsDemo(); awtGraphicsDemo.setVisible(true); } private void prepareGUI(){ setSize(400,400); addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); } @Override public void paint(Graphics g) { Line2D shape = new Line2D.Double(); shape.setLine(250D,250D,150D,150D); Graphics2D g2 = (Graphics2D) g; g2.draw (shape); Font font = new Font("Serif", Font.PLAIN, 24); g2.setFont(font); g.drawString("Welcome to TutorialsPoint", 50, 70); g2.drawString("Line2D.Line", 100, 120); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AWTGraphicsDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AWTGraphicsDemoVerify the following output
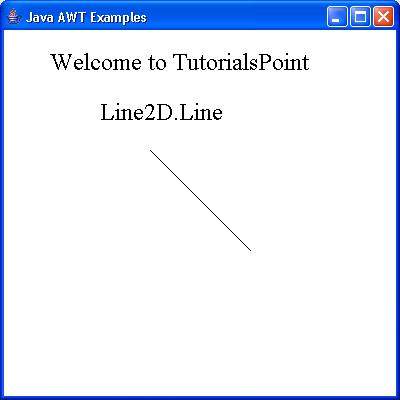
AWT Font Class
Introduction
The Font class states fonts, which are used to render text in a visible way.Class declaration
Following is the declaration for java.awt.Font class:public class Font extends Object implements Serializable
Field
Following are the fields for java.awt.geom.Arc2D class:- static int BOLD -- The bold style constant.
- static int CENTER_BASELINE --The baseline used in ideographic scripts like Chinese, Japanese, and Korean when laying out text.
- static String DIALOG --A String constant for the canonical family name of the logical font "Dialog".
- static String DIALOG_INPUT --A String constant for the canonical family name of the logical font "DialogInput".
- static int HANGING_BASELINE -- The baseline used in Devanigiri and similar scripts when laying out text.
- static int ITALIC -- The italicized style constant.
- static int LAYOUT_LEFT_TO_RIGHT -- A flag to layoutGlyphVector indicating that text is left-to-right as determined by Bidi analysis.
- static int LAYOUT_NO_LIMIT_CONTEXT -- A flag to layoutGlyphVector indicating that text in the char array after the indicated limit should not be examined.
- static int LAYOUT_NO_START_CONTEXT -- A flag to layoutGlyphVector indicating that text in the char array before the indicated start should not be examined.
- static int LAYOUT_RIGHT_TO_LEFT -- A flag to layoutGlyphVector indicating that text is right-to-left as determined by Bidi analysis.
- static String MONOSPACED -- A String constant for the canonical family name of the logical font "Monospaced".
- protected String name -- The logical name of this Font, as passed to the constructor.
- static int PLAIN --The plain style constant.
- protected float pointSize -- The point size of this Font in float.
- static int ROMAN_BASELINE --The baseline used in most Roman scripts when laying out text.
- static String SANS_SERIF -- A String constant for the canonical family name of the logical font "SansSerif".
- static String SERIF -- A String constant for the canonical family name of the logical font "Serif".
- protected int size --The point size of this Font, rounded to integer.
- protected int style -- The style of this Font, as passed to the constructor.
- static int TRUETYPE_FONT -- Identify a font resource of type TRUETYPE.
- static int TYPE1_FONT -- Identify a font resource of type TYPE1.
Class constructors
S.N. | Constructor & Description |
---|---|
1 | protected Font() () Creates a new Font from the specified font. |
2 | Font(Map<? extends AttributedCharacterIterator.Attribute,?> attributes) Creates a new Font from the specified font. |
3 | Font(String name, int style, int size) Creates a new Font from the specified font. |
Class methods
S.N. | Method & Description |
---|---|
1 | boolean canDisplay(char c) Checks if this Font has a glyph for the specified character. |
2 | boolean canDisplay(int codePoint) Checks if this Font has a glyph for the specified character. |
3 | int canDisplayUpTo(char[] text, int start, int limit) Indicates whether or not this Font can display the characters in the specified text starting at start and ending at limit. |
4 | int canDisplayUpTo(CharacterIterator iter, int start, int limit) Indicates whether or not this Font can display the text specified by the iter starting at start and ending at limit. |
5 | int canDisplayUpTo(String str) Indicates whether or not this Font can display a specified String. |
6 | static Font createFont(int fontFormat, File fontFile) Returns a new Font using the specified font type and the specified font file. |
7 | static Font createFont(int fontFormat, InputStream fontStream) Returns a new Font using the specified font type and input data. |
8 | GlyphVector createGlyphVector(FontRenderContext frc, char[] chars) Creates a GlyphVector by mapping characters to glyphs one-to-one based on the Unicode cmap in this Font. |
9 | GlyphVector createGlyphVector(FontRenderContext frc, CharacterIterator ci) Creates a GlyphVector by mapping the specified characters to glyphs one-to-one based on the Unicode cmap in this Font. |
10 | GlyphVector createGlyphVector(FontRenderContext frc, int[] glyphCodes) Creates a GlyphVector by mapping characters to glyphs one-to-one based on the Unicode cmap in this Font. |
11 | GlyphVector createGlyphVector(FontRenderContext frc, String str) Creates a GlyphVector by mapping characters to glyphs one-to-one based on the Unicode cmap in this Font. |
12 | static Font decode(String str) Returns the Font that the str argument describes. |
13 | Font deriveFont(AffineTransform trans) Creates a new Font object by replicating the current Font object and applying a new transform to it. |
14 | Font deriveFont(float size) Creates a new Font object by replicating the current Font object and applying a new size to it. |
15 | Font deriveFont(int style) Creates a new Font object by replicating the current Font object and applying a new style to it. |
16 | Font deriveFont(int style, AffineTransform trans) Creates a new Font object by replicating this Font object and applying a new style and transform. |
17 | Font deriveFont(int style, float size) Creates a new Font object by replicating this Font object and applying a new style and size. |
18 | Font deriveFont(Map<? extends AttributedCharacterIterator.Attribute,?> attributes) Creates a new Font object by replicating the current Font object and applying a new set of font attributes to it. |
19 | boolean equals(Object obj) Compares this Font object to the specified Object. |
20 | protected void finalize() Disposes the native Font object. |
21 | Map<TextAttribute,?> getAttributes() Returns a map of font attributes available in this Font. |
22 | AttributedCharacterIterator.Attribute[] getAvailableAttributes() Returns the keys of all the attributes supported by this Font. |
23 | byte getBaselineFor(char c) Returns the baseline appropriate for displaying this character. |
24 | String getFamily() Returns the family name of this Font. |
25 | String getFamily(Locale l) Returns the family name of this Font, localized for the specified locale. |
26 | static Font getFont(Map<? extends AttributedCharacterIterator.Attribute,?> attributes) Returns a Font appropriate to the attributes. |
27 | static Font getFont(String nm) Returns a Font object fom the system properties list. |
28 | static Font getFont(String nm, Font font) Gets the specified Font from the system properties list. |
29 | String getFontName() Returns the font face name of this Font. |
30 | String getFontName(Locale l) Returns the font face name of the Font, localized for the specified locale. |
31 | float getItalicAngle() Returns the italic angle of this Font. |
32 | LineMetrics getLineMetrics(char[] chars, int beginIndex, int limit, FontRenderContext frc) Returns a LineMetrics object created with the specified arguments. |
33 | LineMetrics getLineMetrics(CharacterIterator ci, int beginIndex, int limit, FontRenderContext frc) Returns a LineMetrics object created with the specified arguments. |
34 | LineMetrics getLineMetrics(String str, FontRenderContext frc) Returns a LineMetrics object created with the specified String and FontRenderContext. |
35 | LineMetrics getLineMetrics(String str, int beginIndex, int limit, FontRenderContext frc) Returns a LineMetrics object created with the specified arguments. |
36 | Rectangle2D getMaxCharBounds(FontRenderContext frc) Returns the bounds for the character with the maximum bounds as defined in the specified FontRenderContext. |
37 | int getMissingGlyphCode() Returns the glyphCode which is used when this Font does not have a glyph for a specified unicode code point. |
38 | String getName() Returns the logical name of this Font. |
39 | int getNumGlyphs() Returns the number of glyphs in this Font. |
40 | java.awt.peer.FontPeer getPeer() Deprecated. Font rendering is now platform independent. |
41 | String getPSName() Returns the postscript name of this Font. |
42 | int getSize() Returns the point size of this Font, rounded to an integer. |
43 | float getSize2D() Returns the point size of this Font in float value. |
44 | Rectangle2D getStringBounds(char[] chars, int beginIndex, int limit, FontRenderContext frc) Returns the logical bounds of the specified array of characters in the specified FontRenderContext. |
45 | Rectangle2D getStringBounds(CharacterIterator ci, int beginIndex, int limit, FontRenderContext frc) Returns the logical bounds of the characters indexed in the specified CharacterIterator in the specified FontRenderContext. |
46 | Rectangle2D getStringBounds(String str, FontRenderContext frc) Returns the logical bounds of the specified String in the specified FontRenderContext. |
47 | Rectangle2D getStringBounds(String str, int beginIndex, int limit, FontRenderContext frc) Returns the logical bounds of the specified String in the specified FontRenderContext. |
48 | int getStyle() Returns the style of this Font. |
49 | AffineTransform getTransform() Returns a copy of the transform associated with this Font. |
50 | int hashCode() Returns a hashcode for this Font. |
51 | boolean hasLayoutAttributes() Return true if this Font contains attributes that require extra layout processing. |
52 | boolean hasUniformLineMetrics() Checks whether or not this Font has uniform line metrics. |
53 | boolean isBold() Indicates whether or not this Font object's style is BOLD. |
54 | boolean isItalic() Indicates whether or not this Font object's style is ITALIC. |
55 | boolean isPlain() Indicates whether or not this Font object's style is PLAIN. |
56 | boolean isTransformed() Indicates whether or not this Font object has a transform that affects its size in addition to the Size attribute. |
57 | GlyphVector layoutGlyphVector(FontRenderContext frc, char[] text, int start, int limit, int flags) Returns a new GlyphVector object, performing full layout of the text if possible. |
58 | String toString() Converts this Font object to a String representation. |
Methods inherited
This class inherits methods from the following classes:- java.lang.Object
Font Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AWTGraphicsDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; import java.awt.geom.*; public class AWTGraphicsDemo extends Frame { public AWTGraphicsDemo(){ super("Java AWT Examples"); prepareGUI(); } public static void main(String[] args){ AWTGraphicsDemo awtGraphicsDemo = new AWTGraphicsDemo(); awtGraphicsDemo.setVisible(true); } private void prepareGUI(){ setSize(400,400); addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); } @Override public void paint(Graphics g) { Graphics2D g2 = (Graphics2D)g; Font plainFont = new Font("Serif", Font.PLAIN, 24); g2.setFont(plainFont); g2.drawString("Welcome to TutorialsPoint", 50, 70); Font italicFont = new Font("Serif", Font.ITALIC, 24); g2.setFont(italicFont); g2.drawString("Welcome to TutorialsPoint", 50, 120); Font boldFont = new Font("Serif", Font.BOLD, 24); g2.setFont(boldFont); g2.drawString("Welcome to TutorialsPoint", 50, 170); Font boldItalicFont = new Font("Serif", Font.BOLD+Font.ITALIC, 24); g2.setFont(boldItalicFont); g2.drawString("Welcome to TutorialsPoint", 50, 220); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AWTGraphicsDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AWTGraphicsDemoVerify the following output
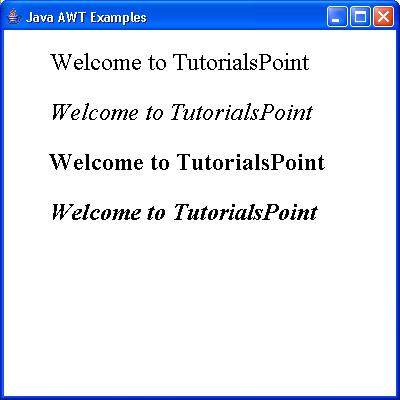
AWT Color Class
Introduction
The Color class states colors in the default sRGB color space or colors in arbitrary color spaces identified by a ColorSpace.Class declaration
Following is the declaration for java.awt.Color class:public class Color extends Object implements Paint, Serializable
Field
Following are the fields for java.awt.geom.Arc2D class:- static Color black -- The color black.
- static Color BLACK -- The color black.
- static Color blue -- The color blue.
- static Color BLUE -- The color blue.
- static Color cyan -- The color cyan.
- static Color CYAN -- The color cyan.
- static Color DARK_GRAY -- The color dark gray.
- static Color darkGray -- The color dark gray.
- static Color gray -- The color gray.
- static Color GRAY -- The color gray.
- static Color green -- The color green.
- static Color GREEN -- The color green.
- static Color LIGHT_GRAY -- The color light gray.
- static Color lightGray -- The color light gray.
- static Color magenta -- The color magenta.
- static Color MAGENTA -- The color magenta.
- static Color orange -- The color orange.
- static Color ORANGE -- The color orange.
- static Color pink -- The color pink.
- static Color PINK -- The color pink.
- static Color red -- The color red.
- static Color RED -- The color red.
- static Color white -- The color white.
- static Color WHITE -- The color white.
- static Color yellow -- The color yellow.
- static Color YELLOW -- The color yellow.
Class constructors
S.N. | Constructor & Description |
---|---|
1 | Color(ColorSpace cspace, float[] components, float alpha) Creates a color in the specified ColorSpace with the color components specified in the float array and the specified alpha. |
2 | Color(float r, float g, float b) Creates an opaque sRGB color with the specified red, green, and blue values in the range (0.0 - 1.0). |
3 | Color(float r, float g, float b, float a) Creates an sRGB color with the specified red, green, blue, and alpha values in the range (0.0 - 1.0). |
4 | Color(int rgb) Creates an opaque sRGB color with the specified combined RGB value consisting of the red component in bits 16-23, the green component in bits 8-15, and the blue component in bits 0-7. |
5 | Color(int rgba, boolean hasalpha) Creates an sRGB color with the specified combined RGBA value consisting of the alpha component in bits 24-31, the red component in bits 16-23, the green component in bits 8-15, and the blue component in bits 0-7. |
6 | Color(int r, int g, int b) Creates an opaque sRGB color with the specified red, green, and blue values in the range (0 - 255). |
7 | Color(int r, int g, int b, int a) Creates an sRGB color with the specified red, green, blue, and alpha values in the range (0 - 255). |
Class methods
S.N. | Method & Description |
---|---|
1 | Color brighter() Creates a new Color that is a brighter version of this Color. |
2 | PaintContext createContext(ColorModel cm, Rectangle r, Rectangle2D r2d, AffineTransform xform, RenderingHints hints) Creates and returns a PaintContext used to generate a solid color pattern. |
3 | Color darker() Creates a new Color that is a darker version of this Color. |
4 | static Color decode(String nm) Converts a String to an integer and returns the specified opaque Color. |
5 | boolean equals(Object obj) Determines whether another object is equal to this Color. |
6 | int getAlpha() Returns the alpha component in the range 0-255. |
7 | int getBlue() Returns the blue component in the range 0-255 in the default sRGB space. |
8 | static Color getColor(String nm) Finds a color in the system properties. |
9 | static Color getColor(String nm, Color v) Finds a color in the system properties. |
10 | static Color getColor(String nm, int v) Finds a color in the system properties. |
11 | float[] getColorComponents(ColorSpace cspace, float[] compArray) Returns a float array containing only the color components of the Color in the ColorSpace specified by the cspace parameter. |
12 | float[] getColorComponents(float[] compArray) Returns a float array containing only the color components of the Color, in the ColorSpace of the Color. |
13 | ColorSpace getColorSpace() Returns the ColorSpace of this Color. |
14 | float[] getComponents(ColorSpace cspace, float[] compArray) Returns a float array containing the color and alpha components of the Color, in the ColorSpace specified by the cspace parameter. |
15 | float[] getComponents(float[] compArray) Returns a float array containing the color and alpha components of the Color, in the ColorSpace of the Color. |
16 | int getGreen() Returns the green component in the range 0-255 in the default sRGB space. |
17 | static Color getHSBColor(float h, float s, float b) Creates a Color object based on the specified values for the HSB color model. |
18 | int getRed() Returns the red component in the range 0-255 in the default sRGB space. |
19 | int getRGB() Returns the RGB value representing the color in the default sRGB ColorModel. |
20 | float[] getRGBColorComponents(float[] compArray) Returns a float array containing only the color components of the Color, in the default sRGB color space. |
21 | float[] getRGBComponents(float[] compArray) Returns a float array containing the color and alpha components of the Color, as represented in the default sRGB color space. |
22 | int getTransparency() Returns the transparency mode for this Color. |
23 | int hashCode() Computes the hash code for this Color. |
24 | static int HSBtoRGB(float hue, float saturation, float brightness) Converts the components of a color, as specified by the HSB model, to an equivalent set of values for the default RGB model. |
25 | static float[] RGBtoHSB(int r, int g, int b, float[] hsbvals) Converts the components of a color, as specified by the default RGB model, to an equivalent set of values for hue, saturation, and brightness that are the three components of the HSB model. |
26 | String toString() Returns a string representation of this Color. |
Methods inherited
This class inherits methods from the following classes:- java.lang.Object
Color Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AWTGraphicsDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; import java.awt.geom.*; public class AWTGraphicsDemo extends Frame { public AWTGraphicsDemo(){ super("Java AWT Examples"); prepareGUI(); } public static void main(String[] args){ AWTGraphicsDemo awtGraphicsDemo = new AWTGraphicsDemo(); awtGraphicsDemo.setVisible(true); } private void prepareGUI(){ setSize(400,400); addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); } @Override public void paint(Graphics g) { Graphics2D g2 = (Graphics2D)g; Font plainFont = new Font("Serif", Font.PLAIN, 24); g2.setFont(plainFont); g2.setColor(Color.red); g2.drawString("Welcome to TutorialsPoint", 50, 70); g2.setColor(Color.GRAY); g2.drawString("Welcome to TutorialsPoint", 50, 120); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AWTGraphicsDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AWTGraphicsDemoVerify the following output
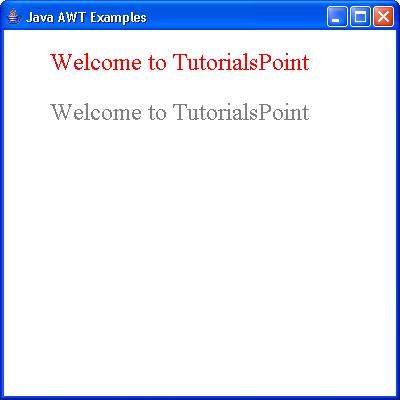
AWT BasicStroke Class
Introduction
The BasicStroke class states colors in the default sRGB color space or colors in arbitrary color spaces identified by a ColorSpace.Class declaration
Following is the declaration for java.awt.BasicStroke class:public class BasicStroke extends Object implements Stroke
Field
Following are the fields for java.awt.geom.Arc2D class:- static int CAP_BUTT -- Ends unclosed subpaths and dash segments with no added decoration.
- static int CAP_ROUND -- Ends unclosed subpaths and dash segments with a round decoration that has a radius equal to half of the width of the pen.
- static int CAP_SQUARE -- Ends unclosed subpaths and dash segments with a square projection that extends beyond the end of the segment to a distance equal to half of the line width.
- static int JOIN_BEVEL -- Joins path segments by connecting the outer corners of their wide outlines with a straight segment.
- static int JOIN_MITER -- Joins path segments by extending their outside edges until they meet.
- static int JOIN_ROUND -- Joins path segments by rounding off the corner at a radius of half the line width.
Class constructors
S.N. | Constructor & Description |
---|---|
1 | BasicStroke() Constructs a new BasicStroke with defaults for all attributes. |
2 | BasicStroke(float width) Constructs a solid BasicStroke with the specified line width and with default values for the cap and join styles. |
3 | BasicStroke(float width, int cap, int join) Constructs a solid BasicStroke with the specified attributes. |
4 | BasicStroke(float width, int cap, int join, float miterlimit) Constructs a solid BasicStroke with the specified attributes. |
5 | BasicStroke(float width, int cap, int join, float miterlimit, float[] dash, float dash_phase) Constructs a new BasicStroke with the specified attributes. |
Class methods
S.N. | Method & Description |
---|---|
1 | Shape createStrokedShape(Shape s) Returns a Shape whose interior defines the stroked outline of a specified Shape. |
2 | boolean equals(Object obj) Tests if a specified object is equal to this BasicStroke by first testing if it is a BasicStroke and then comparing its width, join, cap, miter limit, dash, and dash phase attributes with those of this BasicStroke. |
3 | float[] getDashArray() Returns the array representing the lengths of the dash segments. |
4 | float getDashPhase() Returns the current dash phase. |
5 | int getEndCap() Returns the end cap style. |
6 | int getLineJoin() Returns the line join style. |
7 | float getLineWidth() Returns the line width. |
8 | float getMiterLimit() Returns the limit of miter joins. |
9 | int hashCode() Returns the hashcode for this stroke. |
Methods inherited
This class inherits methods from the following classes:- java.lang.Object
BasicStroke Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AWTGraphicsDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; import java.awt.geom.*; public class AWTGraphicsDemo extends Frame { public AWTGraphicsDemo(){ super("Java AWT Examples"); prepareGUI(); } public static void main(String[] args){ AWTGraphicsDemo awtGraphicsDemo = new AWTGraphicsDemo(); awtGraphicsDemo.setVisible(true); } private void prepareGUI(){ setSize(400,400); addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); } @Override public void paint(Graphics g) { Graphics2D g2 = (Graphics2D)g; g2.setStroke(new BasicStroke(3.0f)); g2.setPaint(Color.blue); Rectangle2D shape = new Rectangle2D.Float(); shape.setFrame(100, 150, 200,100); g2.draw(shape); Rectangle2D shape1 = new Rectangle2D.Float(); shape1.setFrame(110, 160, 180,80); g2.setStroke(new BasicStroke(1.0f)); g2.draw(shape1); Font plainFont = new Font("Serif", Font.PLAIN, 24); g2.setFont(plainFont); g2.setColor(Color.DARK_GRAY); g2.drawString("TutorialsPoint", 130, 200); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtGraphicsDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtGraphicsDemoVerify the following output
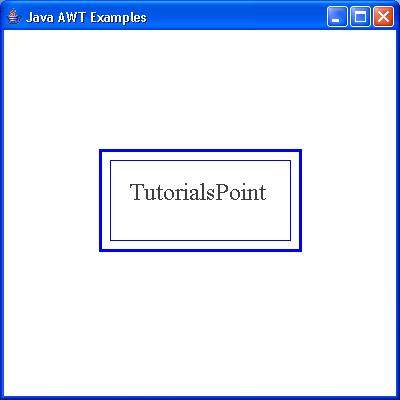
No comments:
Post a Comment