Methods inherited
This class inherits methods from the following classes:- java.awt.Component
- java.lang.Object
List Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtControlDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtControlDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtControlDemo(){ prepareGUI(); } public static void main(String[] args){ AwtControlDemo awtControlDemo = new AwtControlDemo(); awtControlDemo.showListDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showListDemo(){ headerLabel.setText("Control in action: List"); final List fruitList = new List(4,false); fruitList.add("Apple"); fruitList.add("Grapes"); fruitList.add("Mango"); fruitList.add("Peer"); final List vegetableList = new List(4,true); vegetableList.add("Lady Finger"); vegetableList.add("Onion"); vegetableList.add("Potato"); vegetableList.add("Tomato"); Button showButton = new Button("Show"); showButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String data = "Fruits Selected: " + fruitList.getItem(fruitList.getSelectedIndex()); data += ", Vegetables selected: "; for(String vegetable:vegetableList.getSelectedItems()){ data += vegetable + " "; } statusLabel.setText(data); } }); controlPanel.add(fruitList); controlPanel.add(vegetableList); controlPanel.add(showButton); mainFrame.setVisible(true); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtControlDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtControlDemoVerify the following output
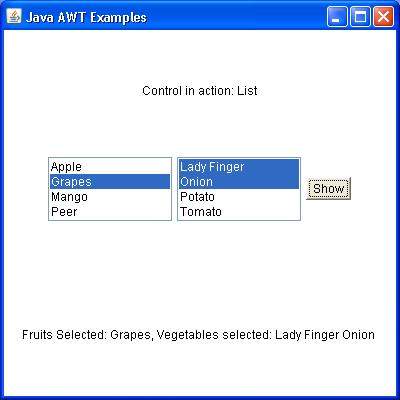
AWT TextField Class
Introduction
The textField component allows the user to edit single line of text.When the user types a key in the text field the event is sent to the TextField. The key event may be key pressed, Key released or key typed. The key event is passed to the registered KeyListener. It is also possible to for an ActionEvent if the ActionEvent is enabled on the textfield then ActionEvent may be fired by pressing the return key.Class declaration
Following is the declaration for java.awt.TextField class:public class TextField extends TextComponent
Class constructors
S.N. | Constructor & Description |
---|---|
1 | TextField() Constructs a new text field. |
2 | TextField(int columns) Constructs a new empty text field with the specified number of columns. |
3 | TextField(String text) Constructs a new text field initialized with the specified text. |
4 | TextField(String text, int columns) Constructs a new text field initialized with the specified text to be displayed, and wide enough to hold the specified number of columns. |
Class methods
S.N. | Method & Description |
---|---|
1 | void addActionListener(ActionListener l) Adds the specified action listener to receive action events from this text field. |
2 | void addNotify() Creates the TextField's peer. |
3 | boolean echoCharIsSet() Indicates whether or not this text field has a character set for echoing. |
4 | AccessibleContext getAccessibleContext() Gets the AccessibleContext associated with this TextField. |
5 | ActionListener[] getActionListeners() Returns an array of all the action listeners registered on this textfield. |
6 | int getColumns() Gets the number of columns in this text field. |
7 | char getEchoChar() Gets the character that is to be used for echoing. |
8 | <T extends EventListener> T[] getListeners(Class<T> listenerType) Returns an array of all the objects currently registered as FooListeners upon this TextField. |
9 | Dimension getMinimumSize() Gets the minumum dimensions for this text field. |
10 | Dimension getMinimumSize(int columns) Gets the minumum dimensions for a text field with the specified number of columns. |
11 | Dimension getPreferredSize() Gets the preferred size of this text field. |
12 | Dimension getPreferredSize(int columns) Gets the preferred size of this text field with the specified number of columns. |
13 | Dimension minimumSize() Deprecated. As of JDK version 1.1, replaced by getMinimumSize(). |
14 | Dimension minimumSize(int columns) Deprecated. As of JDK version 1.1, replaced by getMinimumSize(int). |
15 | protected String paramString() Returns a string representing the state of this TextField. |
16 | Dimension preferredSize() Deprecated. As of JDK version 1.1, replaced by getPreferredSize(). |
17 | Dimension preferredSize(int columns) Deprecated. As of JDK version 1.1, replaced by getPreferredSize(int). |
18 | protected void processActionEvent(ActionEvent e) Processes action events occurring on this text field by dispatching them to any registered ActionListener objects. |
19 | protected void processEvent(AWTEvent e) Processes events on this text field. |
20 | void removeActionListener(ActionListener l) Removes the specified action listener so that it no longer receives action events from this text field. |
21 | void setColumns(int columns) Sets the number of columns in this text field. |
22 | void setEchoChar(char c) Sets the echo character for this text field. |
23 | void setEchoCharacter(char c) Deprecated. As of JDK version 1.1, replaced by setEchoChar(char). |
24 | void setText(String t) Sets the text that is presented by this text component to be the specified text. |
Methods inherited
This class inherits methods from the following classes:- java.awt.TextComponent
- java.awt.Component
- java.lang.Object
TextField Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtControlDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtControlDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtControlDemo(){ prepareGUI(); } public static void main(String[] args){ AwtControlDemo awtControlDemo = new AwtControlDemo(); awtControlDemo.showTextFieldDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showTextFieldDemo(){ headerLabel.setText("Control in action: TextField"); Label namelabel= new Label("User ID: ", Label.RIGHT); Label passwordLabel = new Label("Password: ", Label.CENTER); final TextField userText = new TextField(6); final TextField passwordText = new TextField(6); passwordText.setEchoChar('*'); Button loginButton = new Button("Login"); loginButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String data = "Username: " + userText.getText(); data += ", Password: " + passwordText.getText(); statusLabel.setText(data); } }); controlPanel.add(namelabel); controlPanel.add(userText); controlPanel.add(passwordLabel); controlPanel.add(passwordText); controlPanel.add(loginButton); mainFrame.setVisible(true); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtControlDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtControlDemoVerify the following output
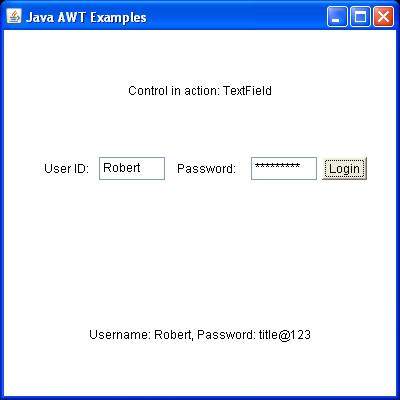
AWT TextArea Class
Introduction
The TextArea control in AWT provide us multiline editor area. The user can type here as much as he wants. When the text in the text area become larger than the viewable area the scroll bar is automatically appears which help us to scroll the text up & down and right & left.Class declaration
Following is the declaration for java.awt.TextArea class:public class TextArea extends TextComponent
Field
Following are the fields for java.awt.TextArea class:- static int SCROLLBARS_BOTH -- Create and display both vertical and horizontal scrollbars.
- static int SCROLLBARS_HORIZONTAL_ONLY -- Create and display horizontal scrollbar only.
- static int SCROLLBARS_NONE -- Do not create or display any scrollbars for the text area.
- static int SCROLLBARS_VERTICAL_ONLY -- Create and display vertical scrollbar only.
Class constructors
S.N. | Constructor & Description |
---|---|
1 | TextArea() Constructs a new text area with the empty string as text. |
2 | TextArea(int rows, int columns) Constructs a new text area with the specified number of rows and columns and the empty string as text. |
3 | TextArea(String text) Constructs a new text area with the specified text. |
4 | TextArea(String text, int rows, int columns) Constructs a new text area with the specified text, and with the specified number of rows and columns. |
5 | TextArea(String text, int rows, int columns, int scrollbars) Constructs a new text area with the specified text, and with the rows, columns, and scroll bar visibility as specified. |
Class methods
S.N. | Method & Description |
---|---|
1 | void addNotify() Creates the TextArea's peer. |
2 | void append(String str) Appends the given text to the text area's current text. |
3 | void appendText(String str) Deprecated. As of JDK version 1.1, replaced by append(String). |
4 | AccessibleContext getAccessibleContext() Returns the AccessibleContext associated with this TextArea. |
5 | int getColumns() Returns the number of columns in this text area. |
6 | Dimension getMinimumSize() Determines the minimum size of this text area. |
7 | Dimension getMinimumSize(int rows, int columns) Determines the minimum size of a text area with the specified number of rows and columns. |
8 | Dimension getPreferredSize() Determines the preferred size of this text area. |
9 | Dimension getPreferredSize(int rows, int columns) Determines the preferred size of a text area with the specified number of rows and columns. |
10 | int getRows() Returns the number of rows in the text area. |
11 | int getScrollbarVisibility() Returns an enumerated value that indicates which scroll bars the text area uses. |
12 | void insert(String str, int pos) Inserts the specified text at the specified position in this text area. |
13 | void insertText(String str, int pos) Deprecated. As of JDK version 1.1, replaced by insert(String, int). |
14 | Dimension minimumSize() Deprecated. As of JDK version 1.1, replaced by getMinimumSize(). |
15 | Dimension minimumSize(int rows, int columns) Deprecated. As of JDK version 1.1, replaced by getMinimumSize(int, int). |
16 | protected String paramString() Returns a string representing the state of this TextArea. |
17 | Dimension preferredSize() Deprecated. As of JDK version 1.1, replaced by getPreferredSize(). |
18 | Dimension preferredSize(int rows, int columns) Deprecated. As of JDK version 1.1, replaced by getPreferredSize(int, int). |
19 | void replaceRange(String str, int start, int end) Replaces text between the indicated start and end positions with the specified replacement text. |
20 | void replaceText(String str, int start, int end) Deprecated. As of JDK version 1.1, replaced by replaceRange(String, int, int). |
21 | void setColumns(int columns) Sets the number of columns for this text area. |
22 | void setRows(int rows) Sets the number of rows for this text area. |
Methods inherited
This class inherits methods from the following classes:- java.awt.TextComponent
- java.awt.Component
- java.lang.Object
TextArea Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AwtControlDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtControlDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtControlDemo(){ prepareGUI(); } public static void main(String[] args){ AwtControlDemo awtControlDemo = new AwtControlDemo(); awtControlDemo.showTextAreaDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showTextAreaDemo(){ headerLabel.setText("Control in action: TextArea"); Label commentlabel= new Label("Comments: ", Label.RIGHT); final TextArea commentTextArea = new TextArea("This is a AWT tutorial " +"to make GUI application in Java.",5,30); Button showButton = new Button("Show"); showButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { statusLabel.setText( commentTextArea.getText()); } }); controlPanel.add(commentlabel); controlPanel.add(commentTextArea); controlPanel.add(showButton); mainFrame.setVisible(true); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtControlDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtControlDemoVerify the following output
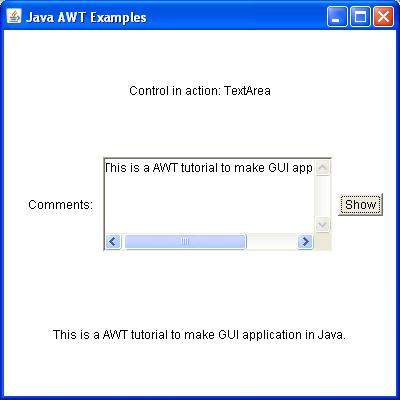
AWT Choice Class
Introduction
Choice control is used to show pop up menu of choices. Selected choice is shown on the top of the menu.Class declaration
Following is the declaration for java.awt.Choice class:public class Choice extends Component implements ItemSelectable, Accessible
Class constructors
S.N. | Constructor & Description |
---|---|
1 | Choice() () Creates a new choice menu. |
Class methods
S.N. | Method & Description |
---|---|
1 | void add(String item) Adds an item to this Choice menu. |
2 | void addItem(String item) Obsolete as of Java 2 platform v1.1. |
3 | void addItemListener(ItemListener l) Adds the specified item listener to receive item events from this Choice menu. |
4 | void addNotify() Creates the Choice's peer. |
5 | int countItems() Deprecated. As of JDK version 1.1, replaced by getItemCount(). |
6 | AccessibleContext getAccessibleContext() Gets the AccessibleContext associated with this Choice. |
7 | String getItem(int index) Gets the string at the specified index in this Choice menu. |
8 | int getItemCount() Returns the number of items in this Choice menu. |
9 | ItemListener[] getItemListeners() Returns an array of all the item listeners registered on this choice. |
10 | <T extends EventListener> T[] getListeners(Class<T> listenerType) Returns an array of all the objects currently registered as FooListeners upon this Choice. |
11 | int getSelectedIndex() Returns the index of the currently selected item. |
12 | String getSelectedItem() Gets a representation of the current choice as a string. |
13 | Object[] getSelectedObjects() Returns an array (length 1) containing the currently selected item. |
14 | void insert(String item, int index) Inserts the item into this choice at the specified position. |
15 | protected String paramString() Returns a string representing the state of this Choice menu. |
16 | protected void processEvent(AWTEvent e) Processes events on this choice. |
17 | protected void processItemEvent(ItemEvent e) Processes item events occurring on this Choice menu by dispatching them to any registered ItemListener objects. |
18 | void remove(int position) Removes an item from the choice menu at the specified position. |
19 | void remove(String item) Removes the first occurrence of item from the Choice menu. |
20 | void removeAll() Removes all items from the choice menu. |
21 | void removeItemListener(ItemListener l) Removes the specified item listener so that it no longer receives item events from this Choice menu. |
22 | void select(int pos) Sets the selected item in this Choice menu to be the item at the specified position. |
23 | void select(String str) Sets the selected item in this Choice menu to be the item whose name is equal to the specified string. |
No comments:
Post a Comment