AWT Graphics Class
Introduction
The Graphics class is the abstract super class for all graphics contexts which allow an application to draw onto components that can be realized on various devices, or onto off-screen images as well.A Graphics object encapsulates all state information required for the basic rendering operations that Java supports. State information includes the following properties.
- The Component object on which to draw.
- A translation origin for rendering and clipping coordinates.
- The current clip.
- The current color.
- The current font.
- The current logical pixel operation function.
- The current XOR alternation color
Class declaration
Following is the declaration for java.awt.Graphics class:public abstract class Graphics extends Object
Class constructors
S.N. | Constructor & Description |
---|---|
1 | Graphics() () Constructs a new Graphics object. |
Class methods
S.N. | Method & Description |
---|---|
1 | abstract void clearRect(int x, int y, int width, int height) Clears the specified rectangle by filling it with the background color of the current drawing surface. |
2 | abstract void clipRect(int x, int y, int width, int height) Intersects the current clip with the specified rectangle. |
3 | abstract void copyArea(int x, int y, int width, int height, int dx, int dy) Copies an area of the component by a distance specified by dx and dy. |
4 | abstract Graphics create() Creates a new Graphics object that is a copy of this Graphics object. |
5 | Graphics create(int x, int y, int width, int height) Creates a new Graphics object based on this Graphics object, but with a new translation and clip area. |
6 | abstract void dispose() Disposes of this graphics context and releases any system resources that it is using. |
7 | void draw3DRect(int x, int y, int width, int height, boolean raised) Draws a 3-D highlighted outline of the specified rectangle. |
8 | abstract void drawArc(int x, int y, int width, int height, int startAngle, int arcAngle) Draws the outline of a circular or elliptical arc covering the specified rectangle. |
9 | void drawBytes(byte[] data, int offset, int length, int x, int y) Draws the text given by the specified byte array, using this graphics context's current font and color. |
10 | void drawChars(char[] data, int offset, int length, int x, int y) Draws the text given by the specified character array, using this graphics context's current font and color. |
11 | abstract boolean drawImage(Image img, int x, int y, Color bgcolor, ImageObserver observer) Draws as much of the specified image as is currently available. |
12 | abstract boolean drawImage(Image img, int x, int y, ImageObserver observer) Draws as much of the specified image as is currently available. |
13 | abstract boolean drawImage(Image img, int x, int y, int width, int height, Color bgcolor, ImageObserver observer) Draws as much of the specified image as has already been scaled to fit inside the specified rectangle. |
14 | abstract boolean drawImage(Image img, int x, int y, int width, int height, ImageObserver observer) Draws as much of the specified image as has already been scaled to fit inside the specified rectangle. |
15 | abstract boolean drawImage(Image img, int dx1, int
dy1, int dx2, int dy2, int sx1, int sy1, int sx2, int sy2, Color
bgcolor, ImageObserver observer) Draws as much of the specified area of the specified image as is currently available, scaling it on the fly to fit inside the specified area of the destination drawable surface. |
16 | abstract boolean drawImage(Image img, int dx1, int
dy1, int dx2, int dy2, int sx1, int sy1, int sx2, int sy2,
ImageObserver observer) Draws as much of the specified area of the specified image as is currently available, scaling it on the fly to fit inside the specified area of the destination drawable surface. |
17 | abstract void drawLine(int x1, int y1, int x2, int y2) Draws a line, using the current color, between the points (x1, y1) and (x2, y2) in this graphics context's coordinate system. |
18 | abstract void drawOval(int x, int y, int width, int height) Draws the outline of an oval. |
19 | abstract void drawPolygon(int[] xPoints, int[] yPoints, int nPoints) Draws a closed polygon defined by arrays of x and y coordinates. |
20 | void drawPolygon(Polygon p) Draws the outline of a polygon defined by the specified Polygon object. |
21 | abstract void drawPolyline(int[] xPoints, int[] yPoints, int nPoints) Draws a sequence of connected lines defined by arrays of x and y coordinates. |
22 | void drawRect(int x, int y, int width, int height) Draws the outline of the specified rectangle. |
23 | abstract void drawRoundRect(int x, int y, int width, int height, int arcWidth, int arcHeight) Draws an outlined round-cornered rectangle using this graphics context's current color. |
24 | abstract void drawString(AttributedCharacterIterator iterator, int x, int y) Renders the text of the specified iterator applying its attributes in accordance with the specification of the TextAttribute class. |
25 | abstract void drawString(String str, int x, int y) Draws the text given by the specified string, using this graphics context's current font and color. |
26 | void fill3DRect(int x, int y, int width, int height, boolean raised) Paints a 3-D highlighted rectangle filled with the current color. |
27 | abstract void fillArc(int x, int y, int width, int height, int startAngle, int arcAngle) Fills a circular or elliptical arc covering the specified rectangle. |
28 | abstract void fillOval(int x, int y, int width, int height) Fills an oval bounded by the specified rectangle with the current color. |
29 | abstract void fillPolygon(int[] xPoints, int[] yPoints, int nPoints) Fills a closed polygon defined by arrays of x and y coordinates. |
30 | void fillPolygon(Polygon p) Fills the polygon defined by the specified Polygon object with the graphics context's current color. |
31 | abstract void fillRect(int x, int y, int width, int height) Fills the specified rectangle. |
32 | abstract void fillRoundRect(int x, int y, int width, int height, int arcWidth, int arcHeight) Fills the specified rounded corner rectangle with the current color. |
33 | void finalize() Disposes of this graphics context once it is no longer referenced. |
34 | abstract Shape getClip() Gets the current clipping area. |
35 | abstract Rectangle getClipBounds() Returns the bounding rectangle of the current clipping area. |
36 | Rectangle getClipBounds(Rectangle r) Returns the bounding rectangle of the current clipping area. |
37 | Rectangle getClipRect() Deprecated. As of JDK version 1.1, replaced by getClipBounds(). |
38 | abstract Color getColor() Gets this graphics context's current color. |
39 | abstract Font getFont() Gets the current font. |
40 | FontMetrics getFontMetrics() Gets the font metrics of the current font. |
41 | abstract FontMetrics getFontMetrics(Font f) Gets the font metrics for the specified font. |
42 | boolean hitClip(int x, int y, int width, int height) Returns true if the specified rectangular area might intersect the current clipping area. |
43 | abstract void setClip(int x, int y, int width, int height) Sets the current clip to the rectangle specified by the given coordinates. |
44 | abstract void setClip(Shape clip) Sets the current clipping area to an arbitrary clip shape. |
45 | abstract void setColor(Color c) Sets this graphics context's current color to the specified color. |
46 | abstract void setFont(Font font) Sets this graphics context's font to the specified font. |
47 | abstract void setPaintMode() Sets the paint mode of this graphics context to overwrite the destination with this graphics context's current color. |
48 | abstract void setXORMode(Color c1) Sets the paint mode of this graphics context to alternate between this graphics context's current color and the new specified color. |
49 | String toString() Returns a String object representing this Graphics object's value. |
50 | abstract void translate(int x, int y) Translates the origin of the graphics context to the point (x, y) in the current coordinate system. |
Methods inherited
This class inherits methods from the following classes:- java.lang.Object
Graphics Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AWTGraphicsDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; import java.awt.geom.*; public class AWTGraphicsDemo extends Frame { public AWTGraphicsDemo(){ super("Java AWT Examples"); prepareGUI(); } public static void main(String[] args){ AWTGraphicsDemo awtGraphicsDemo = new AWTGraphicsDemo(); awtGraphicsDemo.setVisible(true); } private void prepareGUI(){ setSize(400,400); addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); } @Override public void paint(Graphics g) { g.setColor(Color.GRAY); Font font = new Font("Serif", Font.PLAIN, 24); g.setFont(font); g.drawString("Welcome to TutorialsPoint", 50, 150); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AWTGraphicsDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AWTGraphicsDemoVerify the following output
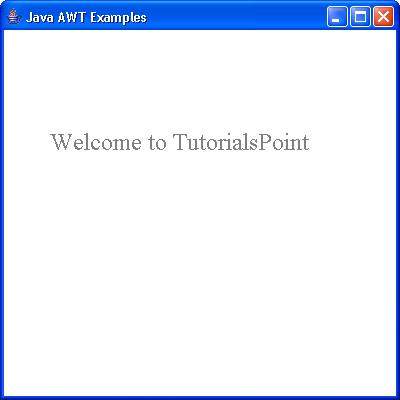
AWT Graphics2D Class
Introduction
The Graphics2D class extends the Graphics class to provide more sophisticated control over geometry, coordinate transformations, color management, and text layout.Class declaration
Following is the declaration for java.awt.Graphics2D class:public abstract class Graphics2D extends Graphics
Class constructors
S.N. | Constructor & Description |
---|---|
1 | Graphics2D() Constructs a new Graphics2D object. |
Class methods
S.N. | Method & Description |
---|---|
1 | abstract void addRenderingHints(Map hints) Sets the values of an arbitrary number of preferences for the rendering algorithms. |
2 | abstract void clip(Shape s) Intersects the current Clip with the interior of the specified Shape and sets the Clip to the resulting intersection. |
3 | abstract void draw(Shape s) Strokes the outline of a Shape using the settings of the current Graphics2D context. |
4 | void draw3DRect(int x, int y, int width, int height, boolean raised) Draws a 3-D highlighted outline of the specified rectangle. |
5 | abstract void drawGlyphVector(GlyphVector g, float x, float y) Renders the text of the specified GlyphVector using the Graphics2D context's rendering attributes. |
6 | abstract void drawImage(BufferedImage img, BufferedImageOp op, int x, int y) Renders a BufferedImage that is filtered with a BufferedImageOp. |
7 | abstract boolean drawImage(Image img, AffineTransform xform, ImageObserver obs) Renders an image, applying a transform from image space into user space before drawing. |
8 | abstract void drawRenderableImage(RenderableImage img, AffineTransform xform) Renders a RenderableImage, applying a transform from image space into user space before drawing. |
9 | abstract void drawRenderedImage(RenderedImage img, AffineTransform xform) Renders a RenderedImage, applying a transform from image space into user space before drawing. |
10 | abstract void drawString(AttributedCharacterIterator iterator, float x, float y) Renders the text of the specified iterator applying its attributes in accordance with the specification of the TextAttribute class. |
11 | abstract void drawString(AttributedCharacterIterator iterator, int x, int y) Renders the text of the specified iterator applying its attributes in accordance with the specification of the TextAttribute class. |
12 | abstract void drawString(String str, float x, float y) Renders the text specified by the specified String, using the current text attribute state in the Graphics2D context. |
13 | abstract void drawString(String str, int x, int y) Renders the text of the specified String, using the current text attribute state in the Graphics2D context. |
14 | abstract void fill(Shape s) Fills the interior of a Shape using the settings of the Graphics2D context. |
15 | void fill3DRect(int x, int y, int width, int height, boolean raised) Paints a 3-D highlighted rectangle filled with the current color. |
16 | abstract Color getBackground() Returns the background color used for clearing a region. |
17 | abstract Composite getComposite() Returns the current Composite in the Graphics2D context. |
18 | abstract GraphicsConfiguration getDeviceConfiguration() Returns the device configuration associated with this Graphics2D. |
19 | abstract FontRenderContext getFontRenderContext() Get the rendering context of the Font within this Graphics2D context. |
20 | abstract Paint getPaint() Returns the current Paint of the Graphics2D context. |
21 | abstract Object getRenderingHint(RenderingHints.Key hintKey) Returns the value of a single preference for the rendering algorithms. |
22 | abstract RenderingHints getRenderingHints() Gets the preferences for the rendering algorithms. |
23 | abstract Stroke getStroke() Returns the current Stroke in the Graphics2D context. |
24 | abstract AffineTransform getTransform() Returns a copy of the current Transform in the Graphics2D context. |
25 | abstract boolean hit(Rectangle rect, Shape s, boolean onStroke) Checks whether or not the specified Shape intersects the specified Rectangle, which is in device space. |
26 | abstract void rotate(double theta) Concatenates the current Graphics2D Transform with a rotation transform. |
27 | abstract void rotate(double theta, double x, double y) Concatenates the current Graphics2D Transform with a translated rotation transform. |
28 | abstract void scale(double sx, double sy) Concatenates the current Graphics2D Transform with a scaling transformation Subsequent rendering is resized according to the specified scaling factors relative to the previous scaling. |
29 | abstract void setBackground(Color color) Sets the background color for the Graphics2D context. |
30 | abstract void setComposite(Composite comp) Sets the Composite for the Graphics2D context. |
31 | abstract void setPaint(Paint paint) Sets the Paint attribute for the Graphics2D context. |
32 | abstract void setRenderingHint(RenderingHints.Key hintKey, Object hintValue) Sets the value of a single preference for the rendering algorithms. |
33 | abstract void setRenderingHints(Map hints) Replaces the values of all preferences for the rendering algorithms with the specified hints. |
34 | abstract void setStroke(Stroke s) Sets the Stroke for the Graphics2D context. |
35 | abstract void setTransform(AffineTransform Tx) Overwrites the Transform in the Graphics2D context. |
36 | abstract void shear(double shx, double shy) Concatenates the current Graphics2D Transform with a shearing transform. |
37 | abstract void transform(AffineTransform Tx) Composes an AffineTransform object with the Transform in this Graphics2D according to the rule last-specified-first-applied. |
38 | abstract void translate(double tx, double ty) Concatenates the current Graphics2D Transform with a translation transform. |
39 | abstract void translate(int x, int y) Translates the origin of the Graphics2D context to the point (x, y) in the current coordinate system. |
Methods inherited
This class inherits methods from the following classes:- java.lang.Object
Graphics2D Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AWTGraphicsDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; import java.awt.geom.*; public class AWTGraphicsDemo extends Frame { public AWTGraphicsDemo(){ super("Java AWT Examples"); prepareGUI(); } public static void main(String[] args){ AWTGraphicsDemo awtGraphicsDemo = new AWTGraphicsDemo(); awtGraphicsDemo.setVisible(true); } private void prepareGUI(){ setSize(400,400); addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); } @Override public void paint(Graphics g) { Graphics2D g2 = (Graphics2D)g; g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON); Font font = new Font("Serif", Font.PLAIN, 24); g2.setFont(font); g2.drawString("Welcome to TutorialsPoint", 50, 70); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AWTGraphicsDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AWTGraphicsDemoVerify the following output
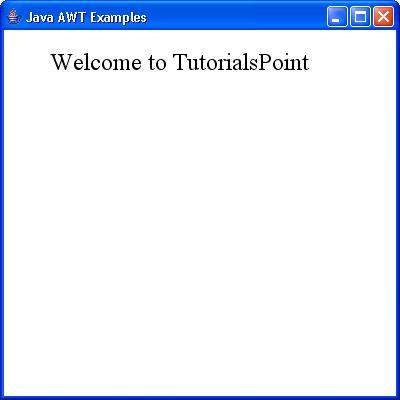
AWT Arc2D Class
Introduction
The Arc2D class is the superclass for all objects that store a 2D arc defined by a framing rectangle, start angle, angular extent (length of the arc), and a closure type (OPEN, CHORD, or PIE).Class declaration
Following is the declaration for java.awt.Arc2D class:public abstract class Arc2D extends RectangularShape
Field
Following are the fields for java.awt.geom.Arc2D class:- static int CHORD -- The closure type for an arc closed by drawing a straight line segment from the start of the arc segment to the end of the arc segment.
- static int OPEN -- The closure type for an open arc with no path segments connecting the two ends of the arc segment.
- static int PIE -- The closure type for an arc closed by drawing straight line segments from the start of the arc segment to the center of the full ellipse and from that point to the end of the arc segment.
Class constructors
S.N. | Constructor & Description |
---|---|
1 | protected Arc2D(int type) This is an abstract class that cannot be instantiated directly. |
Class methods
S.N. | Method & Description |
---|---|
1 | boolean contains(double x, double y) Determines whether or not the specified point is inside the boundary of the arc. |
2 | boolean contains(double x, double y, double w, double h) Determines whether or not the interior of the arc entirely contains the specified rectangle. |
3 | boolean contains(Rectangle2D r) Determines whether or not the interior of the arc entirely contains the specified rectangle. |
4 | boolean containsAngle(double angle) Determines whether or not the specified angle is within the angular extents of the arc. |
5 | boolean equals(Object obj) Determines whether or not the specified Object is equal to this Arc2D. |
6 | abstract double getAngleExtent() Returns the angular extent of the arc. |
7 | abstract double getAngleStart() Returns the starting angle of the arc. |
8 | int getArcType() Returns the arc closure type of the arc: OPEN, CHORD, or PIE. |
9 | Rectangle2D getBounds2D() Returns the high-precision framing rectangle of the arc. |
10 | Point2D getEndPoint() Returns the ending point of the arc. |
11 | PathIterator getPathIterator(AffineTransform at) Returns an iteration object that defines the boundary of the arc. |
12 | Point2D getStartPoint() Returns the starting point of the arc. |
13 | int hashCode() Returns the hashcode for this Arc2D. |
14 | boolean intersects(double x, double y, double w, double h) Determines whether or not the interior of the arc intersects the interior of the specified rectangle. |
15 | protected abstract Rectangle2D makeBounds(double x, double y, double w, double h) Constructs a Rectangle2D of the appropriate precision to hold the parameters calculated to be the framing rectangle of this arc. |
16 | abstract void setAngleExtent(double angExt) Sets the angular extent of this arc to the specified double value. |
17 | void setAngles(double x1, double y1, double x2, double y2) Sets the starting angle and angular extent of this arc using two sets of coordinates. |
18 | void setAngles(Point2D p1, Point2D p2) Sets the starting angle and angular extent of this arc using two points. |
19 | abstract void setAngleStart(double angSt) Sets the starting angle of this arc to the specified double value. |
20 | void setAngleStart(Point2D p) Sets the starting angle of this arc to the angle that the specified point defines relative to the center of this arc. |
21 | void setArc(Arc2D a) Sets this arc to be the same as the specified arc. |
22 | abstract void setArc(double x, double y, double w, double h, double angSt, double angExt, int closure) Sets the location, size, angular extents, and closure type of this arc to the specified double values. |
23 | void setArc(Point2D loc, Dimension2D size, double angSt, double angExt, int closure) Sets the location, size, angular extents, and closure type of this arc to the specified values. |
24 | void setArc(Rectangle2D rect, double angSt, double angExt, int closure) Sets the location, size, angular extents, and closure type of this arc to the specified values. |
25 | void setArcByCenter(double x, double y, double radius, double angSt, double angExt, int closure) Sets the position, bounds, angular extents, and closure type of this arc to the specified values. |
26 | void setArcByTangent(Point2D p1, Point2D p2, Point2D p3, double radius) Sets the position, bounds, and angular extents of this arc to the specified value. |
27 | void setArcType(int type) Sets the closure type of this arc to the specified value: OPEN, CHORD, or PIE. |
28 | void setFrame(double x, double y, double w, double h) Sets the location and size of the framing rectangle of this Shape to the specified rectangular values. |
Methods inherited
This class inherits methods from the following classes:- java.awt.geom.RectangularShape
- java.lang.Object
Arc2D Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AWTGraphicsDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; import java.awt.geom.*; public class AWTGraphicsDemo extends Frame { public AWTGraphicsDemo(){ super("Java AWT Examples"); prepareGUI(); } public static void main(String[] args){ AWTGraphicsDemo awtGraphicsDemo = new AWTGraphicsDemo(); awtGraphicsDemo.setVisible(true); } private void prepareGUI(){ setSize(400,400); addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); } @Override public void paint(Graphics g) { Arc2D.Float arc = new Arc2D.Float(Arc2D.PIE); arc.setFrame(70, 200, 150, 150); arc.setAngleStart(0); arc.setAngleExtent(145); Graphics2D g2 = (Graphics2D) g; g2.setColor(Color.gray); g2.draw(arc); g2.setColor(Color.red); g2.fill(arc); g2.setColor(Color.black); Font font = new Font("Serif", Font.PLAIN, 24); g2.setFont(font); g.drawString("Welcome to TutorialsPoint", 50, 70); g2.drawString("Arc2D.PIE", 100, 120); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtGraphicsDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtGraphicsDemoVerify the following output
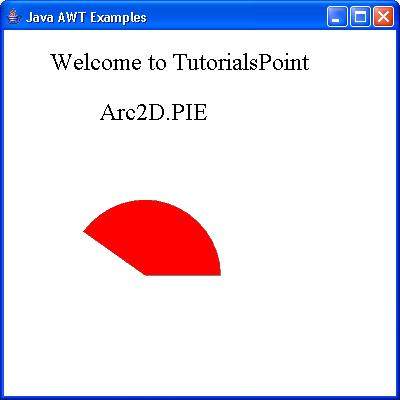
AWT CubicCurve2D Class
Introduction
The CubicCurve2D class states a cubic parametric curve segment in (x,y) coordinate space.Class declaration
Following is the declaration for java.awt.geom.CubicCurve2D class:public abstract class CubicCurve2D extends Object implements Shape, Cloneable
Class constructors
S.N. | Constructor & Description |
---|---|
1 | protected CubicCurve2D() This is an abstract class that cannot be instantiated directly. |
Class methods
S.N. | Method & Description |
---|---|
1 | Object clone() Creates a new object of the same class as this object. |
2 | boolean contains(double x, double y) Tests if the specified coordinates are inside the boundary of the Shape. |
3 | boolean contains(double x, double y, double w, double h) Tests if the interior of the Shape entirely contains the specified rectangular area. |
4 | boolean contains(Point2D p) Tests if a specified Point2D is inside the boundary of the Shape. |
5 | boolean contains(Rectangle2D r) Tests if the interior of the Shape entirely contains the specified Rectangle2D. |
6 | Rectangle getBounds() Returns an integer Rectangle that completely encloses the Shape. |
7 | abstract Point2D getCtrlP1() Returns the first control point. |
8 | abstract Point2D getCtrlP2() Returns the second control point. |
9 | abstract double getCtrlX1() Returns the X coordinate of the first control point in double precision. |
10 | abstract double getCtrlX2() Returns the X coordinate of the second control point in double precision. |
11 | abstract double getCtrlY1() Returns the Y coordinate of the first control point in double precision. |
12 | abstract double getCtrlY2() Returns the Y coordinate of the second control point in double precision. |
13 | double getFlatness() Returns the flatness of this curve. |
14 | static double getFlatness(double[] coords, int offset) Returns the flatness of the cubic curve specified by the control points stored in the indicated array at the indicated index. |
15 | static double getFlatness(double x1, double y1,
double ctrlx1, double ctrly1, double ctrlx2, double ctrly2, double x2,
double y2) Returns the flatness of the cubic curve specified by the indicated control points. |
16 | double getFlatnessSq() Returns the square of the flatness of this curve. |
17 | static double getFlatnessSq(double[] coords, int offset) Returns the square of the flatness of the cubic curve specified by the control points stored in the indicated array at the indicated index. |
18 | static double getFlatnessSq(double x1, double y1,
double ctrlx1, double ctrly1, double ctrlx2, double ctrly2, double x2,
double y2) Returns the square of the flatness of the cubic curve specified by the indicated control points. |
19 | abstract Point2D getP1() Returns the start point. |
20 | abstract Point2D getP2() Returns the end point. |
21 | PathIterator getPathIterator(AffineTransform at) Returns an iteration object that defines the boundary of the shape. |
22 | PathIterator getPathIterator(AffineTransform at, double flatness) Return an iteration object that defines the boundary of the flattened shape. |
23 | abstract double getX1() Returns the X coordinate of the start point in double precision. |
24 | abstract double getX2() Returns the X coordinate of the end point in double precision. |
25 | abstract double getY1() Returns the Y coordinate of the start point in double precision. |
26 | abstract double getY2() Returns the Y coordinate of the end point in double precision. |
27 | boolean intersects(double x, double y, double w, double h) Tests if the interior of the Shape intersects the interior of a specified rectangular area. |
28 | boolean intersects(Rectangle2D r) Tests if the interior of the Shape intersects the interior of a specified Rectangle2D. |
29 | void setCurve(CubicCurve2D c) Sets the location of the end points and control points of this curve to the same as those in the specified CubicCurve2D. |
30 | void setCurve(double[] coords, int offset) Sets the location of the end points and control points of this curve to the double coordinates at the specified offset in the specified array. |
31 | abstract void setCurve(double x1, double y1, double ctrlx1, double ctrly1, double ctrlx2, double ctrly2, double x2, double y2) Sets the location of the end points and control points of this curve to the specified double coordinates. |
32 | void setCurve(Point2D[] pts, int offset) Sets the location of the end points and control points of this curve to the coordinates of the Point2D objects at the specified offset in the specified array. |
33 | void setCurve(Point2D p1, Point2D cp1, Point2D cp2, Point2D p2) Sets the location of the end points and control points of this curve to the specified Point2D coordinates. |
34 | static int solveCubic(double[] eqn) Solves the cubic whose coefficients are in the eqn array and places the non-complex roots back into the same array, returning the number of roots. |
35 | static int solveCubic(double[] eqn, double[] res) Solve the cubic whose coefficients are in the eqn array and place the non-complex roots into the res array, returning the number of roots. |
36 | void subdivide(CubicCurve2D left, CubicCurve2D right) Subdivides this cubic curve and stores the resulting two subdivided curves into the left and right curve parameters. |
37 | static void subdivide(CubicCurve2D src, CubicCurve2D left, CubicCurve2D right) Subdivides the cubic curve specified by the src parameter and stores the resulting two subdivided curves into the left and right curve parameters. |
38 | static void subdivide(double[] src, int srcoff, double[] left, int leftoff, double[] right, int rightoff) Subdivides the cubic curve specified by the coordinates stored in the src array at indices srcoff through (srcoff + 7) and stores the resulting two subdivided curves into the two result arrays at the corresponding indices. |
Methods inherited
This class inherits methods from the following classes:- java.lang.Object
CubicCurve2D Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AWTGraphicsDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; import java.awt.geom.*; public class AWTGraphicsDemo extends Frame { public AWTGraphicsDemo(){ super("Java AWT Examples"); prepareGUI(); } public static void main(String[] args){ AWTGraphicsDemo awtGraphicsDemo = new AWTGraphicsDemo(); awtGraphicsDemo.setVisible(true); } private void prepareGUI(){ setSize(400,400); addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); } @Override public void paint(Graphics g) { CubicCurve2D shape = new CubicCurve2D.Float(); shape.setCurve(250F,250F,20F,90F,140F,100F,350F,330F); Graphics2D g2 = (Graphics2D) g; g2.draw (shape); Font font = new Font("Serif", Font.PLAIN, 24); g2.setFont(font); g.drawString("Welcome to TutorialsPoint", 50, 70); g2.drawString("CubicCurve2D.Curve", 100, 120); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AWTGraphicsDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AWTGraphicsDemoVerify the following output
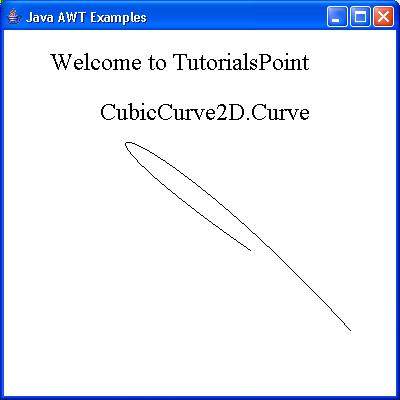
AWT Ellipse2D Class
Introduction
The Ellipse2D class states an ellipse that is defined by a framing rectangle.Class declaration
Following is the declaration for java.awt.geom.Ellipse2D class:public abstract class Ellipse2D extends RectangularShape
Class constructors
S.N. | Constructor & Description |
---|---|
1 | protected Ellipse2D() This is an abstract class that cannot be instantiated directly. |
Class methods
S.N. | Method & Description |
---|---|
1 | boolean contains(double x, double y) Tests if the specified coordinates are inside the boundary of the Shape. |
2 | boolean contains(double x, double y, double w, double h) Tests if the interior of the Shape entirely contains the specified rectangular area. |
3 | boolean equals(Object obj) Determines whether or not the specified Object is equal to this Ellipse2D. |
4 | PathIterator getPathIterator(AffineTransform at) Returns an iteration object that defines the boundary of this Ellipse2D. |
5 | int hashCode() Returns the hashcode for this Ellipse2D. |
6 | boolean intersects(double x, double y, double w, double h) Tests if the interior of the Shape intersects the interior of a specified rectangular area. |
Methods inherited
This class inherits methods from the following classes:- java.lang.Object
Ellipse2D Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >AWTGraphicsDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; import java.awt.geom.*; public class AWTGraphicsDemo extends Frame { public AWTGraphicsDemo(){ super("Java AWT Examples"); prepareGUI(); } public static void main(String[] args){ AWTGraphicsDemo awtGraphicsDemo = new AWTGraphicsDemo(); awtGraphicsDemo.setVisible(true); } private void prepareGUI(){ setSize(400,400); addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); } @Override public void paint(Graphics g) { Ellipse2D shape = new Ellipse2D.Float(); shape.setFrame(100, 150, 200,100); Graphics2D g2 = (Graphics2D) g; g2.draw (shape); Font font = new Font("Serif", Font.PLAIN, 24); g2.setFont(font); g.drawString("Welcome to TutorialsPoint", 50, 70); g2.drawString("Ellipse2D.Oval", 100, 120); } }Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AWTGraphicsDemo.javaIf no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AWTGraphicsDemoVerify the following output
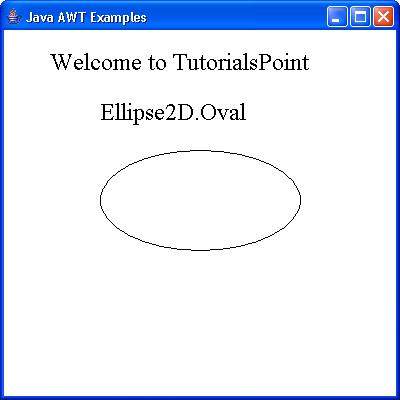
No comments:
Post a Comment